There is no built-in way to comment multiple lines of code in Python like in other languages. Python allows you to comment multiple lines of code by using the #
symbol. This is a great way to make your code more readable and help others understand what you are doing.
Summary: In this article, you’ll learn how to comment multiple lines of code in Python.
Table of Contents
- Why we use Comments in Programming
- Python Single Line Comments
- Python Comment Multiple Lines
- How to create Multiline Comments in Python with VSCode
- Conclusion
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Why we use Comments in Programming
We use comments in programming to help us better understand and document our code. This is especially useful when working with larger or more complex programs, as it allows us to add explanatory notes and reminders about what different parts of the code do. Additionally, comments can be used to disable sections of code temporarily while we are debugging or troubleshooting.
Understanding how to use comments appropriately is a crucial step for learning the Python Syntax.
Python Single Line Comments
In Python, we can use single-line comments by placing the hash symbol (#
) at the start of a line. This tells the interpreter to ignore everything on that line, making it a great way to add notes or reminders about what our code is doing. For example, we might use single-line comments to explain why certain lines of code are necessary or to disable parts of our code temporarily while we are debugging.
To write a single-line comment in Python, simply start the line with a hash symbol (#
):
print("This line gets printed!")
# print("This line doesn't get printed!")
Code language: Python (python)
Output:
This line gets printed!
Code language: plaintext (plaintext)
Python Comment Multiple Lines
While Python does not have a built-in way to comment multiple lines of code like in other languages, we can use multi-line comments by surrounding a block of code with triple quotes ("""
), either starting or ending the line with triple quotes. This tells the interpreter to ignore everything between these lines, making it a great way to add explanatory notes or documentation about our code.
Python Multiline Comments with Triple Quotes
To write multi-line comments in Python, simply surround your code with triple quotes, either starting at the beginning of a line or ending at the end:
"""
print("We don't want any of this...")
print("...to be printed!")
"""
Code language: PHP (php)
Output:
Code language: plaintext (plaintext)
Python Comment Multiple Lines with #
Another way of commenting out multiple lines of Python code is by simply using multiple (#
) in a row:
#print("This should not print!")
#print("Neither should this!")
Code language: Python (python)
Output:
Code language: plaintext (plaintext)
Although Python does not have a built-in way to comment multiple lines of code, using multi-line comments or single-line comments is a great way to add notes and reminders about your code, as well as help others understand what you are doing. Whether you are working on a large or complex program, commenting on your code can be an invaluable tool for improving readability and helping you debug or troubleshoot issues more easily.
How to create Multiline Comments in Python with VSCode
Since a lot of us are using VSCode for Python development, there is a really easy way to comment multiple lines of Python in VSCode!
Comment Multiple Lines of Python in VSCode
- Select the lines you want to comment.
- Press CTRL + # to comment the lines.
Uncomment Multiple Lines of Python in VSCode
- Select the lines you want to uncomment.
- Press CTRL + # to uncomment the lines.
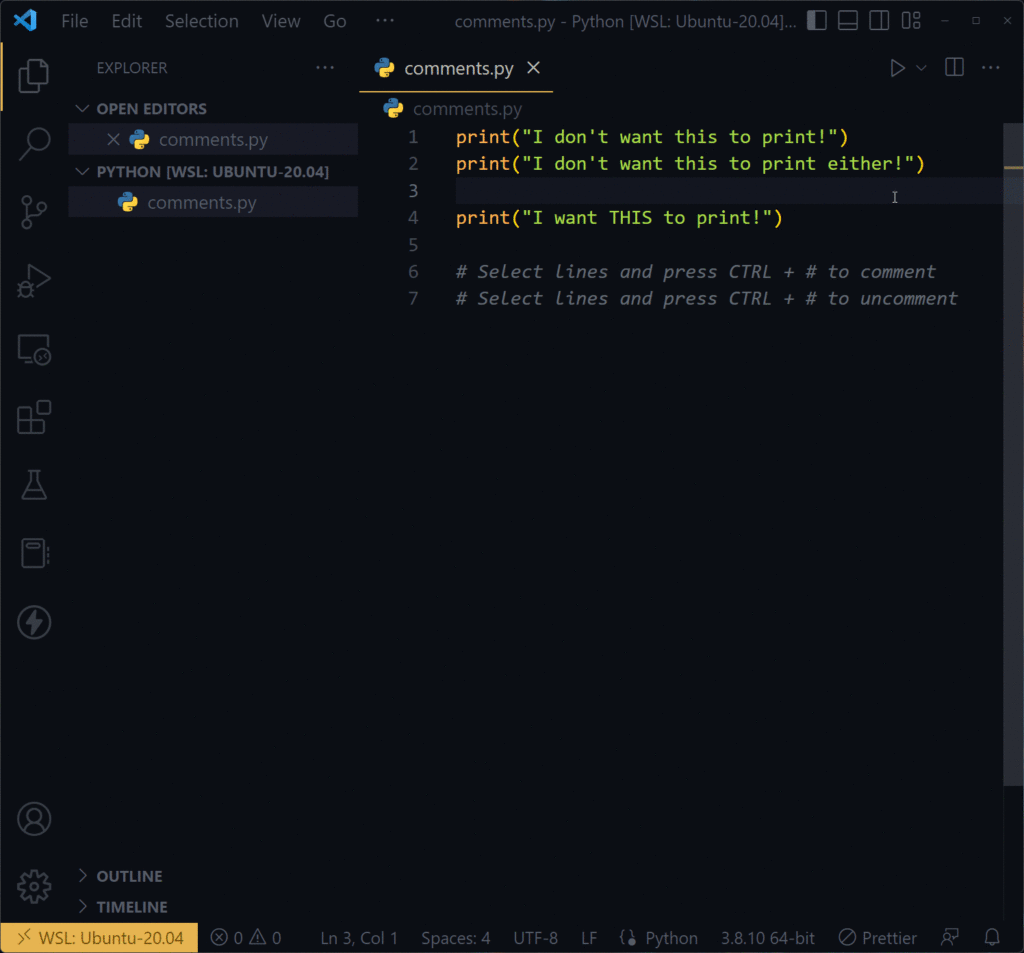
Conclusion
While Python does not have a built-in way to comment multiple lines of code, using multi-line comments or single-line comments is a great way to add notes and reminders about your code, as well as help others understand what you are doing.
Whether you are working on a large or complex program, commenting on your code can be an invaluable tool for improving readability and helping you debug or troubleshoot issues more easily. So if you are looking for an easy way to comment out and uncomment your code in Python, be sure to try out VSCode, and the handy shortcut key for comment/uncomment!
A great way to practice your newly learned information is by creating your very first Python Hello World Program!
To summarize this article:
- You can comment a single line of Python using the
#
syntax. - You can comment multiline comments in Python using triple quotes
""" """
. - You can comment multiline comments in Python using multiple
#
. - The shortcut to comment multiple lines in VSCode is CTRL + #.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection