This tutorial will teach you everything you need to know about Python variables. Variables are among the first things you’ll learn when learning a new programming language. They are an essential part of any language and are widely used to store data.
You can think of a variable as a box with some content. Something where you can store data that you need to use throughout your code.
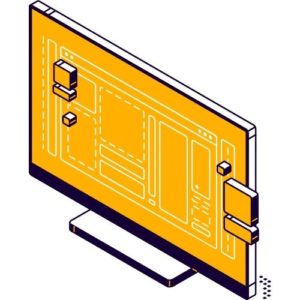
Table of Contents
- Python Variables Basics
- Creating Variables in Python
- Variable Naming Conventions
- Python Variables Summary
Python Variables Basics
The most basic form of a variable that you can create in Python is a variable holding a string value. To declare a variable, you need to assign it a name that is associated with a value. Let’s look at a simple example:
name = "Peter"
hometown = "New York"
print(name)
print(hometown)
Code language: PHP (php)
In the example above, we create a new variable called name
and assign the string "Peter"
to it. We also created another variable called hometown
and assign the string "New York"
to it. You can create a string by either using double or single quotes: "string"
or 'string'
.
The print
function finally prints out these two strings to the console. The result looks like this:
Peter
New York
Code language: plaintext (plaintext)
Creating Variables in Python
As we have seen above, a variable always has a name and a value. To create a variable, we use the following syntax:
variable_name = value
First, we declare the name of the variable, variable_name
, by using the snake case format. Then we utilize the =
sign, which functions as the assignment operator, and finally, we declare the value
, which can be a number, a string, or a boolean.
Variable Naming Conventions
When naming variables in Python, we need to stick to some of the basic rules of Python. It is important that you stick to these rules to avoid errors and that other programmers can read your code:
- You can’t use Python keywords (like
while
orimport
) to declare variables. - Variable names can start with a letter or
_
underscore, but not with a number. - Variable names can contain numbers, but not at the beginning.
- Variables can’t contain spaces (use underscores as a replacement for spaces).
There are also some helpful guidelines that you should stick to. It’s not required, but it will be tremendously helpful when re-reading your own code, or if someone else is working on your code with you:
- Always name variables in a descriptive way. For example,
first_name
is more descriptive than a shortcut likefn
. The same goes for numeric values,person_age = 24
is more descriptive thanx = 24
. - Use underscores instead of spaces to separate words.
Python Variables Summary
Learning how to create variables in Python and learning the basic rules that come with it is a crucial step in learning the Python programming language. Some things to take away are:
- Variables can store data that hold values.
- Use Snake Case to create variable names.
- Variable names should be as descriptive as possible.
- Don’t use numbers at the beginning of variable names.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection