This article will teach you everything you need to know about the Python Input() function. The Python Input() function is one of the most basic Python functions and also one of the things you will learn first when you start learning the Python programming language. But there is more to this function than just capturing user input. We will show you all of the different ways that you can make use of the Python Input() function.
Table of Contents
- What is the Python input() Function?
- Python input() Syntax
- Examples
- Python input() with Integers
- Python input() with Lists
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
What is the Python input() Function?
The input()
function is mainly used to capture user input, but there are more ways in which you can use the function. We will look at all the different use cases for the function below.
Python input() Syntax
The syntax is straightforward:
input(prompt)
Code language: Python (python)
The prompt is optional. It returns any string value you enter to the console as the input message. Once the input()
function executes, it returns the input value to the console.
Examples
Let’s look at a few examples.
Basic Usage
If we simply wrap a string inside of the input()
function, the input()
function gets executed, printing the string value to the console and awaiting user input. After the user enters some input, the input value is also returned to the console:
input("What is your name? ")
Code language: Python (python)
Storing Input in Variables
To make our input()
values reusable and easier to store, we can store user input in variables. This is simple:
print("Enter your name: ")
name = input()
print(f"Hello {name}!")
Code language: Python (python)
First, we use a print()
statement to ask the user for their name, then we create the name
variable and assign it to the input()
function. This prompts the user to enter their name right after the print statement. Finally, we print the user’s name stored inside of the name
variable using an f-string. This is a widely used way of using the input()
function.
Output:
Enter your name:
Stefan
Hello Stefan!
Code language: plaintext (plaintext)
Storing Input in Variables #2
A shorter and more efficient way of achieving the same output as above would be this:
name = input("Enter your name: ")
print(f"Hello {name}!")
Code language: Python (python)
Here, instead of writing a separate print()
statement we utilize the input()
function’s capability to print a prompt to the console. The rest is the same. We store the user input inside of the name
variable and print it to the console using a print()
statement and an f-string.
Output:
Enter your name: Stefan
Hello Stefan!
Code language: plaintext (plaintext)
Storing Input in Variables #3
Of course, you don’t always need to include a print()
statement to capture user input in Python. You can also just declare a variable and assign the input()
function to it. Once the user enters the input, the value is stored in the variable:
name = input()
Code language: Python (python)
Python input() with Integers
We can also use the Python input() function with numbers (integers). To do that, we need to convert the user input to an integer. By default, every input you enter into the input()
function is automatically converted to a string.
The easiest way of doing this is by utilizing Explicit Type Conversion using the built-in int()
function. In the example below, we ask the user for two number inputs and finally, add them together and print the result to the console:
# Getting the first number from a user:
number1 = int(input("Please enter the first number: "))
# Getting the second number from a user:
number2 = int(input("Please enter the second number: "))
# Adding both numbers together and printing the result:
num_result = number1 + number2
print(f"The result is {num_result}")
Code language: Python (python)
Output:
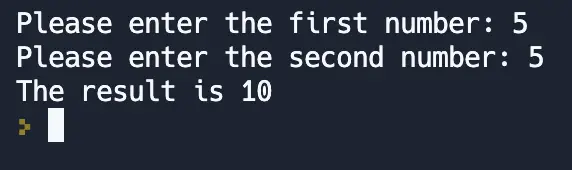
In the example above, we wrap the input()
function inside of the int()
function to convert any input into an integer value. After doing this, we are able to add both of the numbers together and print the result to the console.
Python Input() with Floats
To use a floating point number as an input value, we can utilize the same method by using the built-in float()
function:
# Python input() with Floats
#Getting the first float from a user:
float1 = float(input("Please enter the first float number: "))
#Getting the second float from a user:
float2 = float(input("Please enter the second float number: "))
# Adding both floats together and printing the result:
float_result = float1 + float2
print(f"The result is {float_result}")
Code language: Python (python)
Output:
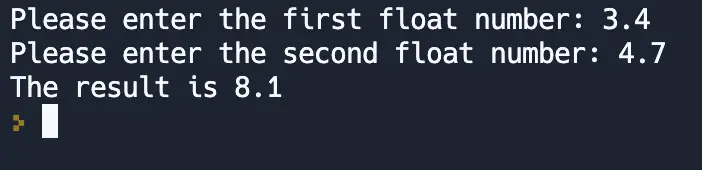
Python input() with Lists
We can also use the Python input() function with lists. This is a good way of prompting a user for list input. In the example below, we prompt the user to input some list values, utilizing yet another Explicit Type Conversion using the built-in list()
function:
# Python input() with lists
# Getting user input and converting it to a list:
user_list = list(input("Add some list elements: "))
print(user_list)
Code language: Python (python)
Output:
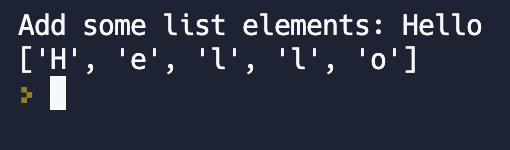
Note that each character of the string “Hello” gets inserted into the list and not the entire string as a whole. The same goes for numbers. If you entered 123
, each number would get inserted into the list separately as a string, not as an int.
Summary
The Python input() function is a powerful way of capturing user input for further processing. If we also utilize Explicit Type Conversion, we can even do mathematical equations with the input the user has provided. Further, we can work with lists as user input. If you want to learn more about Python, make sure to check out our Python category!
- The
input()
function always converts input into a string by default. - Values can be converted to
int()
,float()
andlist()
using Explicit Type Conversion. - Input can be stored in variables for later use.
- A prompt can be used inside of the
input()
function to display text in the console.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection