In this article, you’ll learn everything you know about the built-in Python Filter List function, and we will also show you a few other ways how to filter lists in Python with some practical examples.
Before you learn how to filter list items in Python, you should be familiar with adding items to a list in Python.
Table of Contents
- How do you Filter a List in Python?
- Filter List in Python Using a For Loop
- Filter List in Python using List Comprehension
- Filter List in Python using filter()
- Filter List in Python using Lambda Functions
- List Comprehension vs. Lambda + Filter Performance and Readability
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
How do you Filter a List in Python?
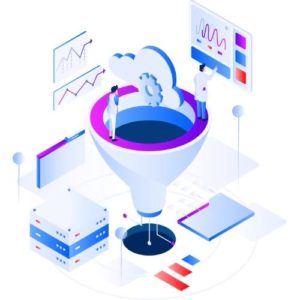
There are three ways how to filter lists in Python:
- Using a for loop.
- Using list comprehension.
- Using the built-in
filter()
function.
Filter List in Python Using a For Loop
The easiest way to Filter a List in Python is by using a for loop. Let’s assume we have a list of some user’s ages, and we want to check which users are over 18 years old:
user_age = [12, 4, 14, 21, 9, 18, 31, 7]
over_eighteen = []
for item in user_age:
if item >= 18:
over_eighteen.append(item)
print(over_eighteen)
Code language: Python (python)
Output:
[21, 18, 31]
Code language: plaintext (plaintext)
How it works
- We initiate an empty list called
over_eighteen
. - We create a for loop that iterates over each item inside of the
user_age
variable and checks if the value is equal to or greater than 18. - The loop appends each item that fits the condition to the empty
over_eighteen
list using the built-inappend()
function.
Filter List in Python using List Comprehension
List Comprehension is basically a shorthand version of the above for loop. List comprehensions allow us to write the same logic as above in one single line of code. Let’s rewrite the for loop in the above’s example into a list comprehension:
user_age = [12, 4, 14, 21, 9, 18, 31, 7]
over_eighteen = [age for age in user_age if age >= 18]
print(over_eighteen)
Code language: Python (python)
Output:
[21, 18, 31]
Code language: plaintext (plaintext)
How it works
- We initiate a list called
over_eighteen
and set it equal to our list comprehension logic. - Inside the list comprehension, we create a for loop and an if statement that iterates over each item in the
user_age
list and check if its value is higher than or equal to 18. If the value is higher or equal to 18, the value is added to theover_eighteen
list.
Python List Comprehension Syntax
The Syntax for list comprehensions is:
new_list = [expression for item in iterable if condition]
Code language: Python (python)
Filter List in Python using filter()
The next method is a bit more complicated than the two methods shown above. In this example, we use the built-in filter()
function to filter items in a list. By default, the filter()
function returns an object, including the filtered elements.
To use the filtered elements in a list, we need to convert the filtered elements into a list using the built-in list()
function.
Let’s use the same user_age
list again in this example:
user_age = [12, 4, 14, 21, 9, 18, 31, 7]
def check_ages(age):
return age >= 18
over_eighteen = filter(check_ages, user_age)
print(list(over_eighteen))
Code language: Python (python)
Output:
[21, 18, 31]
Code language: plaintext (plaintext)
How it works
- We create a function called
check_ages
that checks if the age is greater than or equal to 18 and then returns the result. - Next, we create a variable called
over_eighteen
and assign thefilter()
function to it, passing in ourcheck_ages
function and ouruser_age
list as arguments. - The
filter()
function now iterates over theuser_age
list and executes thecheck_ages
function on each item, returning the values that meet the condition. - Finally, we call the
print()
function and pass in thelist()
function on theover_eighteen
variable to convert it into a Python list.
Python filter() Syntax
The syntax for the filter()
function is:
filter(function, iterable)
Code language: Python (python)
Filter List in Python using Lambda Functions
Lambda functions are functions in Python that do not require a name. They are meant for one-time use. To be able to filter a list in Python using Lambda functions, we also need to utilize the above mentioned filter()
and list()
functions.
To filter lists in Python using Lambda functions, we can use the following syntax:
user_age = [12, 4, 14, 21, 9, 18, 31, 7]
over_eighteen = filter(lambda age: age >= 18, user_age)
print(list(over_eighteen))
Code language: Python (python)
Output:
[21, 18, 31]
Code language: plaintext (plaintext)
How it works
- We create a variable called
over_eighteen
and assign thefilter()
function that carries our Lambda function as an argument to it. - The Lambda function iterates over the
user_age
list and returns each value that meets our condition. - Lambda functions return values per default and don’t need a return keyword.
Python Lambda Syntax
The syntax of Python Lambda functions is:
lambda arguments : expression
Code language: Python (python)
List Comprehension vs. Lambda + Filter Performance and Readability
This is a common question that we get when we discuss the topic of performance in all of the above-mentioned examples. While performance is important, so is readability.
Let’s take these two statements as an example and talk about performance and readability:
Example 1 – List Comprehensions
prices_list = [15, 34, 599, 424, 199, 99]
items_below_hundred_dollars = [price for price in prices_list if price <= 100]
Code language: Python (python)
Example 2 – Lambda + Filter
prices_list = [15, 34, 599, 424, 199, 99]
items_below_hundred_dollars = filter(lambda price: price <= 100, prices_list)
Code language: Python (python)
While it is completely up to personal preference, we think that Example 1, which uses List Comprehensions, is much easier to read than Example 2, which uses the Lambda + Filter functionality.
When it comes to performance, however, the difference is marginal and irrelevant in most cases. While list comprehensions generally perform faster, especially in Python 2, due to the list comprehension only accessing local variables, the difference is mostly not worth the sacrifice of readability.
Do not think in terms of simple code like the examples above. Think in terms of more complex code that uses lambda functions. Things can get squiggly real quick there.
Example 3 – Generator Function
If you really need performance and it is your top priority, creating a generator instead of a list comprehension would probably be wise:
prices_list = [15, 34, 599, 424, 199, 99]
def filterPrices(list, value):
for item in list:
if item.attribute <= value: yield item
Code language: Python (python)
Summary
Now you should have a pretty good understanding of all the different ways you can implement Python Filter List functions in your code. We recommend giving all of them a try and sticking to the one that you like most. If you are looking to replace list items with Python, take a look at this article.
To further summarize:
- There are three ways to filter lists in Python:
- For loops
- List comprehensions
- The built-in
filter()
function
- To Filter a List in Python using a for loop, initiate an empty list and append each item that fits the condition to this empty list.
- List comprehension is a shorthand version of using a for loop and can be written in one line of code.
- The syntax for creating list comprehensions is:
new_list = [expression for item in iterable if condition]
- The built-in
filter()
function returns an object, including the filtered elements. To use these filtered elements as a list, they must first be converted into a list using the built-inlist()
function.- The syntax for
filter ()
is :filter (function , iterable )
- The syntax for
- Lambda functions are functions without names that are meant to be used only once.
- Syntax:
lambda arguments: expression
- Syntax:
- List Comprehension is generally considered to be faster than using the
filter()
or Lamba functions.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection