In this article, you’ll learn everything you need to know about the Python xrange function.
Table of Contents
- What is Python xrange?
- What does xrange mean in Python?
- What is the difference between xrange and range in Python?
- Python xrange Syntax
- Python xrange Examples
- Is xrange faster than range?
- Things To Keep In Mind When Using Python xrange
What is Python xrange?
In Python, the range()
function is a built-in function that returns a sequence of numbers. The range function takes two arguments: start and stop. The start argument is the first number in the sequence. The stop argument is one greater than the last number in the sequence. So, if you want to generate a sequence of numbers from 1 to 10 using the range()
function, you would use this code:
# Python range example
for i in range(1,11):
print(i)
Code language: Python (python)
This code prints out all numbers between 1 and 11, not including 11.
But what if you want to generate a really long sequence of numbers? Let’s say you want to generate a list of all the integers from 1 to 1 billion. Trying to create that list using the range
function would take forever because you would have to specify the stop argument as 1000000000 (that’s 10 followed by 9 zeros). Luckily, there’s a solution: The Python xrange function.
What does xrange mean in Python?
The Python xrange function works by returning an xrange
object that generates a Sequence of Numbers on Demand. So, rather than generate the entire sequence of numbers upfront, which would take forever for really big ranges, it generates them as you need them. That’s why it’s able to handle really large ranges without any problems.
What is the difference between xrange and range in Python?
Python xrange and range are both built-in functions in Python that generate a list of numbers. The main difference between the two is that xrange generates an “xrange object” while range generates a list. An “xrange object” is similar to a list, but instead of storing all the values in memory, it only stores the sequence information.
This is important when dealing with large lists of numbers, as xrange will use less memory than range. Another difference is that xrange is only available in Python 2, while range is available in both Python 2 and 3. However, in Python 3, range is equivalent to xrange. Therefore, if you’re using Python 3, there’s no need to use xrange.
- The
range()
function – Returns a range object (iterable). - The
xrange()
function – Returns the generator which can be used for looping and displaying numbers.
xrange() | range() |
---|---|
Returns generator object. | Returns list of integers. |
Faster. | Slower. |
Uses less memory. | Uses more memory. |
No Python 3 Support. | Python 2 and Python 3 Support |
Python xrange Syntax
The syntax of the Python xrange() function is really simple:
xrange(start, end, step)
Code language: Python (python)
The parameters used here are the following:
- start – Specifies the starting position.
- end – Specifies the ending position.
- step – Specifies the difference between all numbers in a sequence.
While you have to define an end position, the start and the step parameters are optional.
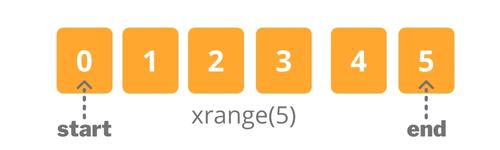
Python xrange Examples
Let’s look at a few examples. Suppose we want to create a list of integers from 1 to 10 using the xrange
function. We would do that like this:
Example 1 – Simple xrange Function
my_list = list(xrange(1, 11))
print(my_list)
Code language: Python (python)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Code language: plaintext (plaintext)
As you can see from the example above, we were able to successfully create a list of integers from 1 to 10 using the xrange
function without any problems. So how does it work under the hood?
Well, when you call list(xrange(1, 11))
, what happens is that an object called an “iterator” is created. That iterator then goes through each number in your specified range one at a time and adds it to your list. Once it reaches the end of your specified range (in our case 11), it stops and returns your completed list.
Example 2 – xrange with end Parameter
Now Let’s look at another example where we define an end parameter:
end = 4
xrange_with_end_parameter = xrange(end)
for number in xrange_with_end_parameter:
print(number)
Code language: PHP (php)
Output:
0
1
2
3
In this example, we define the end position with the number 4. Then, we define the xrange_with_end_parameter
variable and assign it to xrange(end)
.
Finally, we use a simple for loop to print out all of the numbers up until the end position to the console. As you can see, it starts from 0 and all the way up until 3 but not including the end number 4.
Example 3 – xrange with start and end Parameters
Now let’s pick up on the above example, but this time, we also include a start parameter:
start = 2
end = 4
xrange_with_end_parameter = xrange(start, end)
for number in xrange_with_end_parameter:
print(number)
Code language: Python (python)
Output:
2
3
Code language: plaintext (plaintext)
In this example, we define start at number 2 and end at number 4. This means xrange()
starts at position 2 and loops all the way until position 4, printing the numbers to the console.
Example 4 – xrange with start, end, and step Parameters
Let’s put it all together and also include a step parameter:
start = 2
end = 10
step = 2
xrange_with_end_parameter = xrange(start, end, step)
for number in xrange_with_end_parameter:
print(number)
Code language: Python (python)
Output:
2
4
6
8
By adding the step parameter of 2, the xrange()
function now steps two positions with each iteration. As you can see in the output, it always jumps two positions to the next higher number.
Example 5 – range and xrange Type Checking
Let’s look at range()
and xrange()
side by side and check its return types:
# Using the range() function
range_function = range(1,11)
# Using the xrange() function
xrange_function = xrange(1,11)
# Checking types of return values
print ("The range_function return type is: ")
print(type(range_function))
print ("The xrange_function return type is: ")
print(type(xrange_function))
Code language: Python (python)
Output:
The range_function return type is:
<type 'list'>
The xrange_function return type is:
<type 'xrange'>
Code language: plaintext (plaintext)
We can clearly see that the output of the range_function
is a Python list
and the output of the xrange_function
is xrange
, which represents the xrange object.
Is xrange faster than range?
Since the xrange()
function only evaluates the generator object containing the values which are required by lazy evaluation, xrange is faster than range. The range()
function, on the other hand, would be faster if you would iterate over the same sequence of values multiple times. The range()
function, however, will always consume more memory than xrange()
, which means you need to decide on a case-by-case basis which one of the two functions you are going to use.
Things To Keep In Mind When Using Python xrange
Now that we know how Python xrange works and have seen it in action, let’s go over some things you need to keep in mind when using it:
- If you’re working with very large ranges (like 1 billion or more), make sure you use Python xrange instead of range because otherwise, your program will eventually run out of memory and crash.
- You can’t directly index into an “xrange object,” so if you need random access to elements inside a range (like if you’re creating something like a deck of cards), make sure you use Python range instead so that your program doesn’t crash when trying to index into an “xrange object.”
- Keep in mind that xrange objects are only available in Python 2; they don’t exist in Python 3 because their functionality has been rolled into Python’s regular range function (just called “range” instead of “xrange”). So if you’re working with Python 3 code and come across references to “xrange,” just replace them with “range.”
- Use
xrange()
overrange()
if memory usage is a concern.
So there you have it! Now you know all about how Python xrange works and what things you need to keep in mind when using it!
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection