Python Constants are used to indicate that a value assigned to them should not be changed. This makes them ideal for use in mathematical equations or as part of a program’s interface. In this post, we’ll take a look at how to create constants, and we’ll also provide some examples of where they can be useful.
Table of Contents
- What are Constants in Python?
- How do you create a Constant in Python?
- Python Constants can be changed
- Python Constants Naming Conventions
- Constants in Python Examples
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
What are Constants in Python?
Python offers several built-in constants, such as True
, False
, and None
. Python also allows users to create their custom constants using the keyword None.
Constants are stored in memory, and they are accessed using their respective names. Constants can be of any data type, including integers, floats, strings, and tuples. Constants are typically used to represent unchanging values in a Python program, such as the value of pi or the number of days in a week. When creating constants, it is important to choose names that accurately describe their purpose.
Since constants do not officially exist, their value can be changed during program execution. Constants are used to indicate a value that should not be touched if working together with other programmers.
How do you create a Constant in Python?
Constants are created by using capitalized letters separated by underscores (_
). A simple constant with a string value looks like this:
CAPITAL_OF_GERMANY = "Berlin"
Code language: Python (python)
Python Constants can be changed
This means that you can change their value during runtime. Let’s assume we want to change the string value of the CAPITAL_OF_GERMANY
constant from “Berlin” to “Munich” during runtime:
# A constant in Python
CAPITAL_OF_GERMANY = "Berlin"
print(CAPITAL_OF_GERMANY)
# Changing constants in Python is possible
CAPITAL_OF_GERMANY = "Munich"
print(CAPITAL_OF_GERMANY)
Code language: Python (python)
Output:
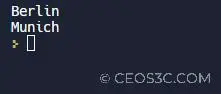
Using constants is merely an indicator for other developers working on your code that certain values should not be changed.
Python Constants Naming Conventions
Constants should always be named in all capital letters separated by underscores (_
). Here are some examples:
MY_HOME_ADDRESS = "Sunnylane 23"
MY_BIRTHYEAR = 1982
BEER_TASTES_GOOD = True
Code language: Python (python)
Constants in Python Examples
Some more examples of all the different use-cases where constants can be used:
Lists
OUR_LIST = ["Apples", "Bananas", "Mangoes"]
Code language: Python (python)
Tuples
OUR_TUPLE = ("Apples", "Bananas", "Mangoes")
Code language: Python (python)
Dictionaries
OUR_DICT = {"Fruit": "Mango", "Vegetable": "Carrot"}
Code language: Python (python)
Summary
To summarize, constants are mainly used to indicate to other developers that certain variables shouldn’t be changed.
- Constants are written with capitalized letters separated by underscores (
_
). - Constants can be changed.
- Constants can be booleans, strings, integers, lists, tuples, and dictionaries, among others.
- We can create as many constants as we like.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection