The Python print() function is one of the first things we learn when learning the Python programming language. As in other programming languages, one of the first things you do is print the line Hello World!
to the console. The same is true for Python.
But, there is more to the Python print() function than simply printing strings or numbers to the console with it. The Python print() function is also used for debugging, among other things. In this article, you will learn everything you need to know about the Python print() function.
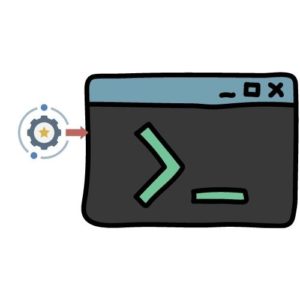
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Table of Contents
- What is the Python print() Function?
- Python print() Syntax
- Python print() Basics
- Formatting Options
- More Python print() Examples
- Debugging with print()
- Python print() Summary
What is the Python print() Function?
The Python print()
function can print values to the console. But why do we need this functionality? There is a multitude of use cases for the print()
function. You can print strings, numbers, boolean values, tuples, objects, and other things to the console.
The print()
function in a Python context is mostly used for debugging but is not limited to this functionality. It is often used in courses and tutorials to build text-based games or exercises to better understand and learn the language. It is important to know the Python print() syntax.
Python print() Syntax
The most basic way of using the print()
function is by printing a string to the console:
print("Hello World!")
Code language: PHP (php)
Output:
Hello World!
Code language: plaintext (plaintext)
Python print() Syntax Breakdown
The full print()
syntax, according to the Python documentation, looks like this:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
Code language: PHP (php)
The following parameters can be used:
- objects – Any object you would like to print. Converted to a string before it is printed.
- sep – Optional: A separator with the default value of
' '
(a space) to separate printed objects. - end – Optional: This allows you to specify what to print at the end of the print statement. The default is a newline character
'\n'
. - file – Optional: This allows you to print an object that has a write method. The default is
sys.stdout
. - flush – Optional: A boolean value that defines if the output is flushed
True
or bufferedFalse
. The default value isFalse
.
While all of this is nice to know, you mostly will not need any of these parameters except for debugging purposes.
Python print() Basics
The print()
function takes one or more objects as arguments, which can be strings, numbers, or other data types. You can separate the objects with commas.
For example, here’s how you can use the print()
function to output a string:
print("Hello, world!")
Code language: Python (python)
This will output the following text to the console:
Hello, world!
Code language: plaintext (plaintext)
Outputting Variables:
You can also use the print()
function to output variables. For example:
name = "Alice"
age = 30
print("My name is", name, "and I am", age, "years old.")
Code language: Python (python)
This will output the following text to the console:
My name is Alice and I am 30 years old.
Code language: plaintext (plaintext)
The sep
and end
Arguments:
You can use the sep
and end
arguments to customize the output of the print()
function. The sep
argument specifies what separator should be used between the objects you’re printing, and the end
argument specifies what character(s) should be used at the end of the output.
For example, here’s how you can use the sep
and end
arguments to output a list of names separated by commas:
names = ["Alice", "Bob", "Charlie"]
print(*names, sep=", ", end=".\\n")
Code language: Python (python)
This will output the following text to the console:
Alice, Bob, Charlie.
Code language: plaintext (plaintext)
These are the basics of the print()
function in Python. With just a few lines of code, you can output text and data to the console, which is an essential part of any Python program. In the next sections, we’ll explore some of the more advanced features of the print()
function, including formatting options and best practices.
Formatting Options
In addition to basic output, the print()
function provides several formatting options that can be used to customize the appearance of the output. Here are some of the most common formatting options:
String Formatting
String formatting is a powerful way to format text in Python. The str.format()
method can be used to insert variables and values into a string, and to format the output in various ways.
For example, here’s how you can use the str.format()
method to format a string with variables:
name = "Alice"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
Code language: Python (python)
This will output the following text to the console:
My name is Alice and I am 30 years old.
Code language: plaintext (plaintext)
You can also use string formatting to specify the width and precision of numeric values, to display values in scientific notation, and more.
F-Strings
F-strings are a newer, more concise way to format strings in Python 3.6 and later. F-strings allow you to embed expressions inside string literals, using curly braces {}
.
For example, here’s how you can use an f-string to format a string with variables:
name = "Alice"
age = 30
print(f"My name is {name} and I am {age} years old.")
Code language: Python (python)
This will output the same text as the previous example:
My name is Alice and I am 30 years old.
Code language: plaintext (plaintext)
F-strings are generally considered more readable and easier to use than other formatting options.
Padding and Alignment
You can use padding and alignment options to format output in columns or tables. For example, here’s how you can use the str.format()
method to align text in columns:
names = ["Alice", "Bob", "Charlie"]
ages = [30, 25, 40]
for name, age in zip(names, ages):
print("{:<10} {:>5}".format(name, age))
Code language: Python (python)
This will output the following text to the console:
Alice 30
Bob 25
Charlie 40
The :<10
and :>5
parts of the format string specify the width and alignment of the columns.
These are just a few of the formatting options available for the print()
function in Python. By using these options, you can create more complex and sophisticated output that meets your specific needs.
More Python print() Examples
To give you a better idea of how the print()
function works, let’s look at some more real-world examples. We already learned how to print "Hello World"
to the console, as the most basic form of using the print()
statement.
Printing Python Lists
We can print lists using the print()
function. For this, we must first create a list and then print it to the console:
our_list = ["Python", "JavaScript", "Golang"]
print(our_list)
Code language: PHP (php)
What do you think will be printed? Only the string values or the entire list?
Output:
['Python', 'JavaScript', 'Golang']
Code language: plaintext (plaintext)
Naturally, the entire list will be printed. To be able to print out separate values of a list, we need to use a loop.
Printing a String using a Separator
We can use the sep
parameter we have learned about earlier to separate two strings:
print("Hi!", "How was your day so far?", sep="+++")
Code language: PHP (php)
Output:
Hi!+++How was your day so far?
The separator will be inserted at the location of the comma ,
after the string "Hi!"
. This can be useful in some cases.
Printing Using the File Parameter
Another parameter we can use is the file
parameter. This allows us to open certain files in writing mode. In this example, we attempt to open a file called file.txt using our code. Of the file does not exist, Python will create it for us and opens it in writing mode:
our_file = open("file.txt", "w")
print("This is the content of our file.txt file!", file = our_file)
our_file.close()
Code language: PHP (php)
The file.txt file should have been created on your system with the content of our print()
statement.
Note: This even works on Replit. You have to open the Shell and type:
cat file.txt
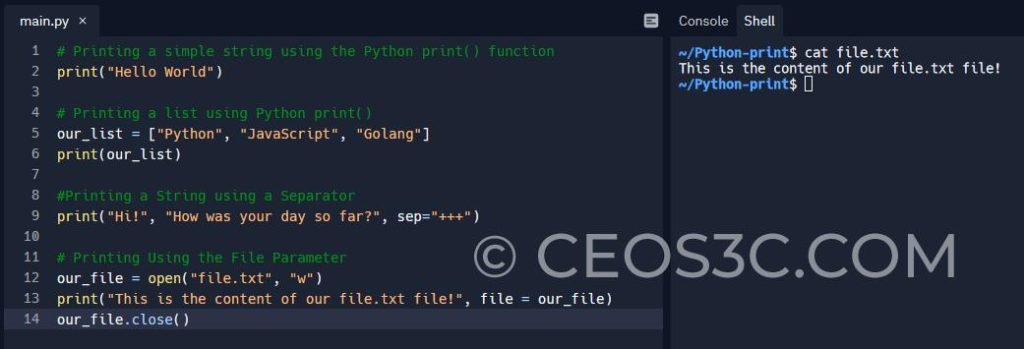
Debugging with print()
Let’s look at some examples of how to use Python print()
for debugging purposes. Using the print() function for debugging is only one way of debugging your Python code. Using print() to debug your code is also known as print debugging, and it is the most basic form of debugging.
To find errors in your code using the print()
function, you want to include print()
statements throughout your code or in specific places where you suspect the error happens. This helps you to identify wrong or falsy results when running your code easily.
Let’s assume we want to print a string if number2
is larger than number1
, and we have mixed up our numbers:
number1 = 5
number2 = 3
if number2 > number1:
print("number2 is definitely larger than number1!")
Code language: PHP (php)
If we run our code, we don’t get any output because number2
is, in fact, smaller than number1
. Now just assume this is a more complicated piece of code with potentially many more lines. The easiest approach would be to include print()
statements underneath all the potential variables that our code requires to run:
number1 = 5
print("number1 = ", number1)
number2 = 3
print("number2 = ", number2)
if number2 > number1:
print("number2 is definitely larger than number1!")
Code language: PHP (php)
Looking at the output of this code, we would clearly see that number1
is larger than number2
.
Output:
number1 = 5
number2 = 3
This example should give you a pretty good idea of how we can use the print()
function for debugging purposes.
Python print() Summary
These are just a few examples of the power of the Python print() function. You should definitely internalize this function as you will certainly need it for debugging your code at some point. It is a powerful tool to go through each line of your code and analyze it for errors.
If you want to learn more about Python in a beginner-friendly way, make sure to bookmark our Python Tutorials section or check out some of the related tutorials below!
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection
I watched a Kali Linux video one time and now it seems to be the only video that populates when trying to take these python courses. There doesn’t seem to be a pattern that I can recognize yet and It doesn’t happen every time but I will try to document what I notice and comment in as much detail as I can. Keep up the good work I love these clear and concise videos!