In today’s article, you’ll learn everything you need to know about enum in Python. Enum is a shorthand for enumeration in Python. In Python, we create enumerations using classes that include names (members) bound to unique values. The values are constant.
Table of Contents
- What is Enum used for in Python?
- How to use Enum in Python?
- Python Enum Examples
- Enumerations in Python are Immutable
- Summary
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
What is Enum used for in Python?
Enum in Python is used for iteration and comparison of the names (members) you assign to the class. We can use a for loop to iterate over all of the members of the enum class. But be aware that while we use the class syntax for enums, enums are not treated as typical Python classes.
How to use Enum in Python?
There is a multitude of ways how to use Enum in Python. The easiest way to learn is by working through the examples below. In general, the enum class is used by importing Enum
and then creating a class with Enum
as a parameter and some enum members:
from enum import Enum
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
Python Enum Examples
The best way to understand how Enum in Python works is by working through some examples together. You can fork the Repl to play around with it as we go!
Example 1 – The Python Enum Class
Here is an example of what the enum class looks like and what it can return:
from enum import Enum
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
# Print enum member as string
print(CarBrands.BMW)
# print name of enum member using 'name' keyword
print(CarBrands.BMW.name)
# print value of enum member using the 'value' keyword
print(CarBrands.BMW.value)
# print type of enum member using the type() function
print(type(CarBrands.BMW))
# print enum member as repr
print(repr(CarBrands.BMW))
# print all enum members using the "list" keyword
print(list(CarBrands))
Code language: Python (python)
Output:
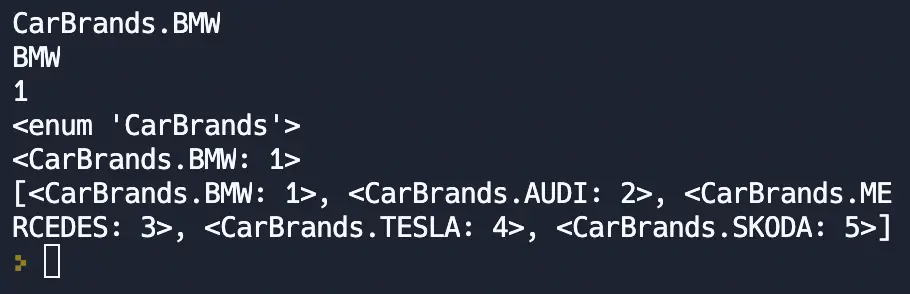
Example 2 – Accessing Enum Member Data
To access data of enum members, we can target them either by value or name:
- Use the
value
keyword to access a member’s value. - Use the
name
keyword to access a member’s name.
Here is an example of how we would use this method on some of our example data:
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
# getting name of enum member using 'name' keyword
print(f"The name of the enum member with the value of 1 is: {CarBrands(1).name}")
# getting value of enum member using the 'value' keyword
print(f"The value of the enum member with the name of 'BMW' is: {CarBrands['BMW'].value}")
Code language: Python (python)
Output:

Enum members are callable. This allows us to get an enum member by its value
. We can try accessing the car brand TESLA
by its value 4
:
print(CarBrands(4))
Code language: PHP (php)
Output:
CarBrands.TESLA
Code language: CSS (css)
The Enum class also uses the __getitem__
method, which we can utilize by using square brackets to access an enum member by its name.
We are able to get the member TESLA
by its name using this syntax:
print(CarBrands['TESLA']))
Code language: CSS (css)
Output:
CarBrands.TESLA
Code language: CSS (css)
Example 3 – Iterating Enums using For Loops
As briefly mentioned above, we can iterate through enum members using for loops. This allows us to print out all of the members of the enum class. As you can see in the example below, we can also include keywords like name
and value
in the for loop to display data in a more structured way:
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
# Using a for loop to iterate through enum class members
for cars in (CarBrands):
print(f"{cars.value} - {cars.name}")
Output:
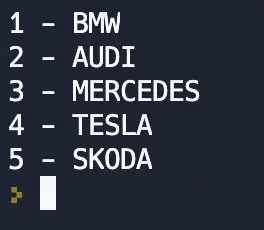
Example 4 – Working with Hashes
Next, we look at how we can work with hashes using our enum members to be able to work with dictionaries and sets. The first version where we use our_dict
is using a simple if/else block to check if the values are hashed. The second version where we use our_dict_two
is using a shorter, more practical form:
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
# Hashing enum member as dictionary
our_dict = {}
our_dict[CarBrands.BMW] = 'Munich'
our_dict[CarBrands.AUDI] = 'Ingolstadt'
# Evaluating if enum values are hashed or not
if our_dict == {CarBrands.BMW: 'Munich', CarBrands.AUDI: 'Ingolstadt'}:
print("Enum Member is hashed.")
else:
print("Enum Member is not hashed.")
# Shorter version
our_dict_two = {}
our_dict_two == {CarBrands.BMW: 'Munich', CarBrands.AUDI: 'Ingolstadt'}
Code language: Python (python)
Output:
Enum Member is hashed.
Code language: plaintext (plaintext)
Example 5 – Comparing Enumeration Results
We are also able to compare our enumeration results using both, the keywords is
and is not
as well as the comparison operators ==
and !=
.
A good example would be the following:
class CarBrands(Enum):
BMW = 1
AUDI = 2
MERCEDES = 3
TESLA = 4
SKODA = 5
# Comparison using "is and is not"
if CarBrands.BMW is CarBrands.BMW:
print("That's clearly a BMW car!")
else:
print("That's not a BMW car!")
# Comparison using "!= and =="
if CarBrands.BMW == CarBrands.BMW:
print("That's clearly a BMW car!")
else:
print("That's not a BMW car!")
Code language: Python (python)
Output:
That's clearly a BMW car!
That's not a BMW car!
Code language: plaintext (plaintext)
Example 6 – Checking if Member is Present
We can use the in
keyword to check if a certain member is present within the enumeration members or not:
if CarBrands.BMW in CarBrands:
print("The car brand is present!")
else:
print("The car brand is not present!")
Code language: Python (python)
Output:
The car brand is present!
Code language: plaintext (plaintext)
Enumerations in Python are Immutable
This prevents us from adding or removing members once the enumeration is set. This also prevents us from changing member values.
If we want to add a new member to the CarBrands
enumeration, we receive a TypeError
:
CarBrands['Volkswagen'] = 6
Code language: Python (python)
Output:
Traceback (most recent call last):
File "main.py", line 90, in <module>
CarBrands['Volkswagen'] = 6
TypeError: 'EnumMeta' object does not support item assignment
Code language: plaintext (plaintext)
The same is true if we want to change a value of an existing enum member:
CarBrands['BMW'].value = 8
Code language: Python (python)
Output:
Traceback (most recent call last):
File "main.py", line 92, in <module>
CarBrands['BMW'].value = 8
AttributeError: can't set attribute
Code language: plaintext (plaintext)
Summary
Understanding enum in Python can be a bit daunting. The best way to learn it is to work through some of the examples we have provided above and play around with the code. Enum in Python has many different use cases and is a widely used function.
- Enum Member Values can be
int
,str
, orauto
. If the value is not of importance,auto
may be used and the correct value will be chosen automatically. Avoid mixingauto
with other values. - We can display enum members as strings and repr.
- We can use the
type()
function on enum members to check their type. - We can use the
list()
function to get a list of all enum members. - We can access enum member names by using the
name
attribute. - Enum members are hashable, which means we can use them in dictionaries as well as in sets.
- Enum members should always be declared using UPPER_CASE names since they are constants.
- Enumerations in Python are immutable.
- Enumerations in Python are callable.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection
Hello,
Great Enum article! However, I think I found a small problem, if I am reading things right. In Example 5, I think the condition results for != and == and backwards.
# Comparison using “!= and ==”
if CarBrands.BMW != CarBrands.BMW:
print(“That’s clearly a BMW car!”)
else:
print(“That’s not a BMW car!”)
Hope this is helpful!
Mike
Oh man, we definitely messed that one up!
Thank you for pointing that out, it is corrected now! 🙂
We appreciate your feedback. Thanks for reading!