To write a Python Inline if else One Line statement, we need to use the ternary operator (conditional expression). Writing multiple if-else statements in Python is also possible but should be avoided for better readability.
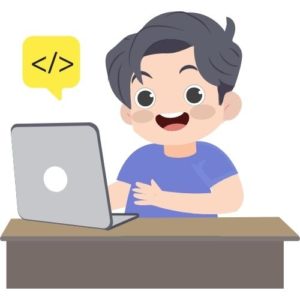
Table of Contents
- Python Inline if else One Line Syntax
- Classic if/else in Python
- How do you write if and else in one line?
- How do you write multiple if-else in one line in Python?
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Python Inline if else One Line Syntax
The syntax to write an inline if else one-liner is:
expression if condition else different_expression
Code language: Python (python)
If you want to inform the user if it’s sunny or if it’s raining, that would look like this:
is_raining = True
weather = "Take an umbrella!" if is_raining else "Take sunglasses!"
Code language: PHP (php)
While using one-line if else statements can shorten your code, it can also make it much harder to read. That is true for anyone who works with you, but it also makes it harder for you to get back into your code if you haven’t worked on it for a while.
Maintaining good readability is crucial, so you have to always consider if using a one-line if else statement in Python makes sense for each case.
Classic if/else in Python
Before we look at some more Python inline if else one line statement examples, let’s look at a classical if/else block:
is_raining = True
if is_raining:
weather_info = "Take an umbrella!"
else:
weather_info = "Take sunglasses!"
print(weather_info)
Code language: Python (python)
Output:
Take an umbrella!
How do you write if and else in one line?
Let’s look at how we can turn this into a one-line if-else statement using the ternary operator:
is_raining = True
weather_info = "Take an umbrella!" if is_raining else "Take sunglasses!"
print(weather_info)
Code language: Python (python)
Output:
Take an umbrella!
How do you write multiple if-else in one line in Python?
You can use the ternary operator to chain together multiple if else statements in one line:
number = 50
output = 10 if number < 10 else 20 if number > 10 else 0
print(output)
Code language: Python (python)
Output:
20
While this works, we do not recommend using this approach. It is very hard to read. A much clearer approach, in this case, would be:
number = 50
if number < 10:
output = 10
elif number > 10:
output = 20
else:
output = 0
print(output)
Code language: Python (python)
Output:
20
Code language: plaintext (plaintext)
Summary
To summarize, while it is possible to use Python inline if else one-line statements, they should be used with caution. If we want to maintain good readability, we should avoid longer one-line statements.
- Python inline if else one line statements can be written using the ternary operator.
- Writing long one-line statements should be avoided for the sake of readability.
- Multiple if-else one-line statements can be written in Python but should be used cautiously.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection