Python list slicing is a process of selecting a specific range of items from a Python list. This can be done by specifying the starting and ending indices or by using Python slice notation. In this tutorial, we will show you how to slice Python lists in different ways and explain the benefits of each approach.
Table of Contents
- Python List Slicing Syntax
- What is the difference between slicing and indexing a list in Python?
- Python List Slicing Examples
- Python List Slicing Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Python List Slicing Syntax
Python list slicing is a process of selecting a certain range of items from a Python list. This can be done by specifying the starting and ending indices or by using Python slice notation.
The most basic form of Python list slicing is specifying the start and end indices of the slice, separated by a colon:
our_list[start:end:IndexJump]
Code language: Python (python)
Positive and Negative Indexes
There are positive and negative indexes in list slicing. Let’s run through both cases together to better illustrate what we mean by that.
Positive Indexes
Let’s use a simple list as an example: our_list = ["Apples", "Oranges", "Mangosteen", "Bananas"]
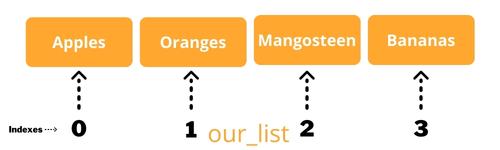
If we want to print out the entire list, we can simply use the following code:
our_list = ["Apples", "Oranges", "Mangosteen", "Bananas"]
print(our_list[::])
Code language: Python (python)
Output:

We can achieve the same output by using the following code:
our_list = ["Apples", "Oranges", "Mangosteen", "Bananas"]
print(our_list[0:4])
Code language: Python (python)
Output:

As you can probably see here, we are using the index of 0 and the index of 4, but our list actually only has 3 indexes (0, 1, 2, 3, 4). We need to use 4 as the end index because list slicing does not include the last index by default, meaning if we would try to use print(our_list[0:3])
, the result would exclude the last index that holds the value of "Bananas"
.
Negative Indexes
If we wanted to print the entire list again using negative indexes, we have to use the following code:
our_list = ["Apples", "Oranges", "Mangosteen", "Bananas"]
print(our_list[-4::1])
Code language: Python (python)
Output:

Here, -4 represents the first index of the list, and 1 represents the last index of the list. The result is exactly the same.
What is the difference between slicing and indexing a list in Python?
Indexing a Python list refers to selecting an individual element from the list. This can be done by using the indexing operator [ ]
with the index of the element you want to select. For example, if we have a Python list called our_list
, we can access the first element of the list like this: our_list[0]
Slicing a Python list, on the other hand, allows you to select a range of elements from the list. This is done by specifying two indices: the starting index and the ending index (up to but not including). For example, if we have a Python list called our_list
, we can slice it like this: our_list[0:100]
. This will select the first 100 elements from the list.
Both indexing and slicing Python lists have their own benefits and use cases. Indexing and slicing Python lists is a fundamental skill that every Python programmer should know.
Python List Slicing Examples
The best way to illustrate Python List Slicing is by using a few examples.
Example 1
Let’s say we wanted to get the values "Oranges"
and "Mangosteen"
printed out from our list using Python List Slicing:
our_list = ["Apples", "Oranges", "Mangosteen", "Bananas"]
print(our_list[1:3])
Code language: Python (python)
Output:
['Oranges', 'Mangosteen']
Code language: plaintext (plaintext)
Example 2
Another good example would be to use a list of numbers, which better illustrates how indexes work:
number_list = [0, 1, 2, 3, 4, 5, 6]
Code language: Python (python)
What if we wanted to print the numbers 0 – 3? We can use this code:
print(number_list[0:4])
Code language: Python (python)
Output:
[0, 1, 2, 3]
Code language: plaintext (plaintext)
Now, what if we wanted to print out every second value of that list? We can do this by using the code:
print(number_list[::2])
Code language: Python (python)
Here we leave the start and end position as default, indicated by the two ::
. Then, we specify an index jump of 2, telling the function to only print every second index.
Output:
[0, 2, 4, 6]
Code language: plaintext (plaintext)
What if we wanted to print out the numbers starting at index 1 and ending with index 5, but we only want to print every third number? We can achieve this by using the code:
print(number_list[1:5:3])
Code language: Python (python)
Output:
[1, 4]
Code language: plaintext (plaintext)
Python List Slicing Summary
This should give you a pretty good idea of how Python List Slicing works. Python List Slicing is a great way of working with lists and can save you a lot of boilerplate code. To summarize:
- Python list slicing refers to selecting a range of items from a Python list.
- This can be done by specifying the starting and ending indices or by using Python slice notation.
- We can define Index Jumps to skip certain indices.
- List slicing is a powerful technique that allows you to select a sublist from a given Python list.
- Indexing a Python list refers to selecting an individual element from the list, while slicing allows you to select a range of elements.
- Both indexing and slicing have their own benefits and use cases. Every Python programmer should know how to both index and slice lists.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection