Python is the rising star in today’s programming landscape. Interesting fields like Machine Learning and Artificial Intelligence are attracting more and more people to the Python programming language daily. This article teaches you how to write your first Python Hello World Program.
Table of Contents
- What is a Hello World Program?
- Python Hello World Syntax
- How to run a Python Hello World Program
- How to create a Python Hello World Program from Scratch
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
What is a Hello World Program?
A Python Hello World program is a short program that prints out the string "Hello World"
to the console. The purpose of a Python Hello World program is to show you how to write and run a Python program. Python is a very popular programming language, and it’s used in many different ways.
Some people use Python for web development, others use it for data science or machine learning, and still, others use it for general scripting or automation. Python is also an excellent language for beginners to learn because it’s relatively simple and easy to read.
Python Hello World Syntax
There are two ways you can print out "Hello World"
to the console using Python Syntax:
- Using the
print()
function - Using the
sys.stdout.write()
function
The most common way you will come across will be the Python print() function. Let’s look at both versions below.
Python Hello World using print()
To print out “Hello World” to the console using the print()
function, we can use the following code:
print("Hello World")
Code language: Python (python)
Output:
Hello World
Code language: plaintext (plaintext)
Python Hello World using sys.stdout.write()
To print out "Hello World"
to the console using the sys.stdout.write()
function. To be able to use sys.stdout.write()
, we need to first import the sys
library that is included in Python. To make it work, we can use the following code:
import sys
sys.stdout.write("Hello World")
Code language: JavaScript (javascript)
Output:
Hello World
Code language: plaintext (plaintext)
How to run a Python Hello World Program
The easiest way to demonstrate how to create a Python Hello World Program is by using a browser-based IDE (Integrated Development Environment) like Replit. Replit is entirely free to use, and you can just fork (copy) our existing code and run it in your browser!
1 – Fork our Repository
Click on the Fork Repl button above or on this link to open our repository. Once this is done, click on the blue Fork Repl button to create your own copy to play around with. You can also just run the code by pressing the big play button in the middle:
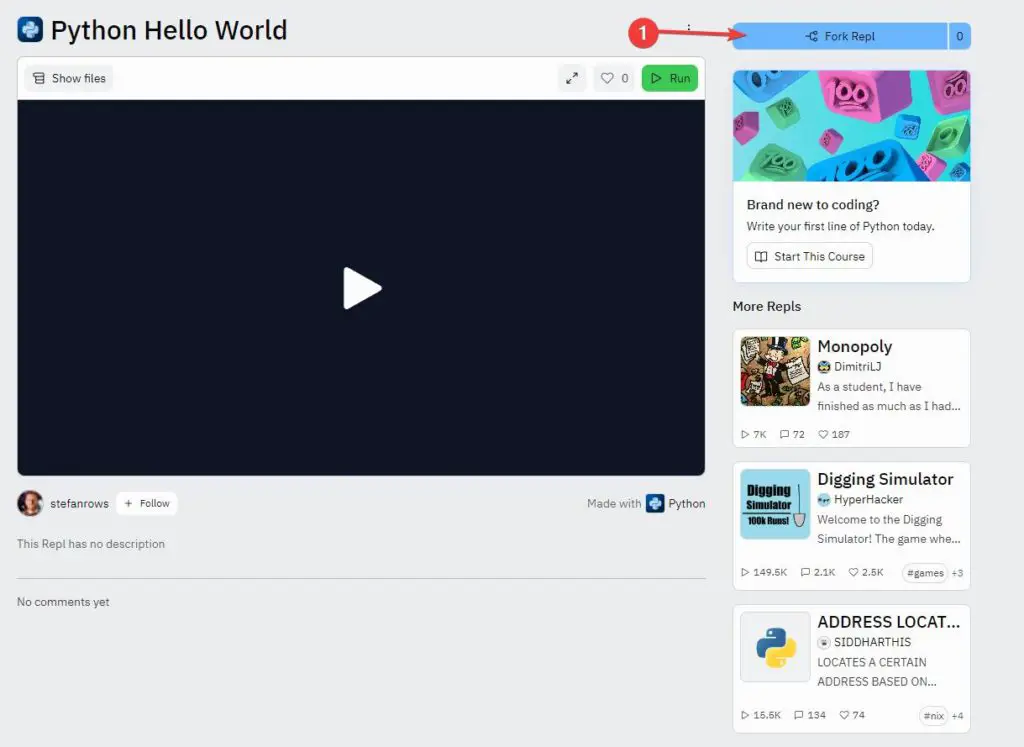
2 – Run the Code
Once you have forked the Repl, you can click on the big green Run button on the top of your screen to run the code. Feel free to change the printed message from "Hello World"
to anything you like, and press the Run button again to see what happens!
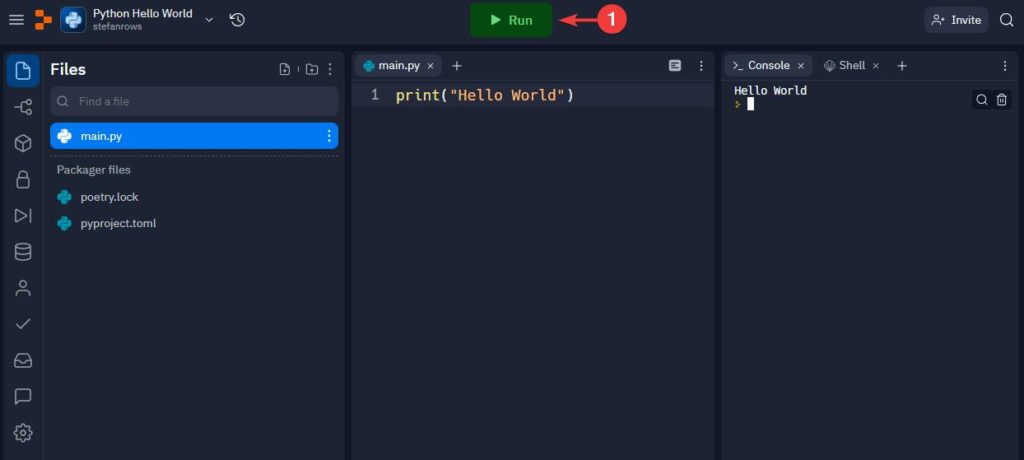
How to create a Python Hello World Program from Scratch
To create a Python Hello World Program from scratch, you need to follow through with a couple more steps.
Installing Python 3
First, you must ensure that Python 3 is installed on your computer. To do that, head over to the Python website and download the latest Python 3 installer.
- For Windows, it’s the Windows installer (64-bit) package.
- For macOS, it’s the macOS 64-bit unversial2 installer.
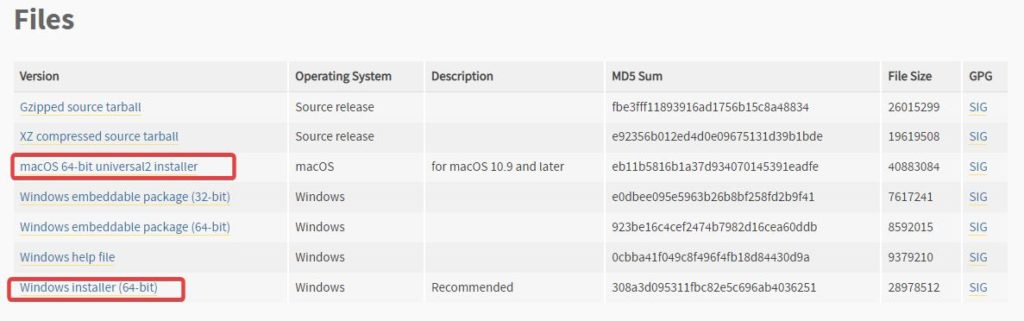
Once downloaded, run the installer. Make sure to click the checkmark for “Add python.exe to PATH” before clicking on Install Now. Everything else can be left as default.
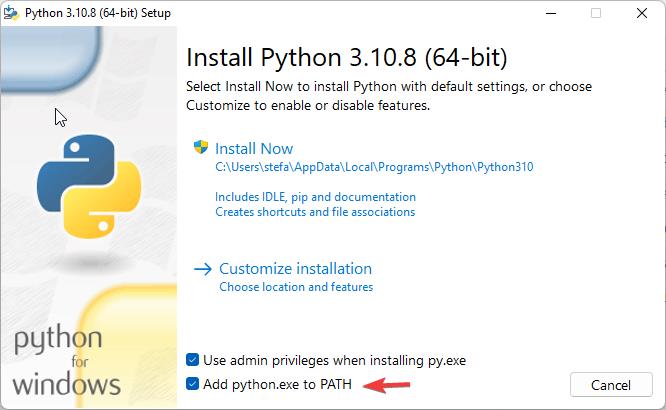
Running the Python Hello World Program in IDLE
Python IDLE is a Python Integrated Development and Learning Environment that is bundled with the default Python installation. It provides a Python Shell window and an editor to write and run Python scripts (also called programs).
To launch Python IDLE, just open your Start Menu, go to the Programs or All Programs folder, and look or search for IDLE. If you just press your Windows key and start typing IDLE, you’ll find it. When Python IDLE launches, you will see the Python Shell window appear. This is where you can write and execute your Python code.
To write a Python Hello World Program, just type the following code into the Python Shell window:
print("Hello World")
Code language: Python (python)
Then, to run the Python program, go to the Run menu at the top of the Python Shell window and select Run Module. Alternatively, you can press F5 on your keyboard or just hit the Enter button.
You should then see "Hello World"
printed out in the Python Shell window:
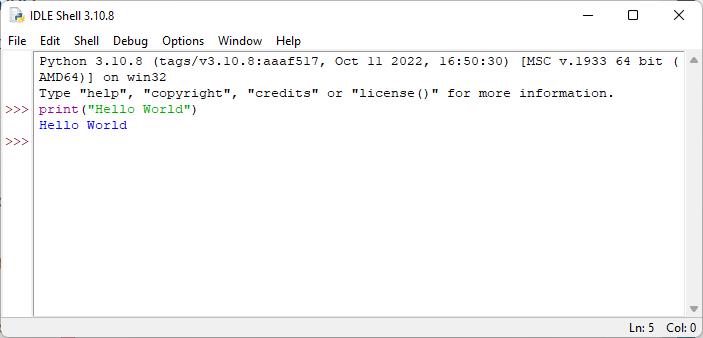
Summary
Python is a versatile language that can be used for building many different types of applications, including web apps, gaming apps, data analysis tools, machine learning algorithms, and more. Python is also a great language for beginners to learn because it’s relatively simple and easy to read.
- There are two ways you can print out
"Hello World"
to the console using Python:print("Hello World")
sys.stdout.write("Hello World")
- You can run a Python Hello World Program by using a browser-based IDE like Replit or by installing Python 3 and running the code in Python IDLE.
Congratulations, you’ve just written your first Python program!
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection