In Python, strings are immutable. This means that you cannot change the contents of a string once it has been created. However, you can use the str append Python function to add new content to an existing string. In this blog post, we will discuss the basics of Python string append and show you how to use it in your own code!
Table of Contents
- Python String Append Syntax
- How to Append one String to Another in Python?
- Python Append String Multiple Times
- Python Append String using the join() Function
- Python Append String using f-strings
- Add one Python String to Another using __add__
- Python Str Append using the format() Function
- Which Method is the Best?
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Python String Append Syntax
The str append Python function allows you to add new content to an existing string. This can be useful if you need to modify the contents of a string dynamically without having to create a new string altogether.
The basic syntax to use Python string append is by using the +
and +=
operators:
our_string = 'Python'
print(our_string)
our_string += ' is'
print(our_string)
our_string += ' very cool!'
print(our_string)
Code language: Python (python)
Output:
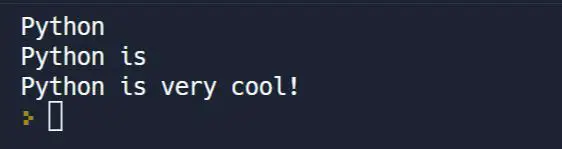
As you can see in the example above, our initial string of Python gets extended every time we use the +=
syntax to concatenate another word to the original string.
How to Append one String to Another in Python?
To append one string to another in Python, we can simply use the +=
operator as shown in the example above. Let’s assume we have an address of Rainbowstreet
and a street number of 1
, both stored in different variables. In the end, we want to put them together. We can use the following syntax to achieve that:
street = "Rainbowstreet"
street_number = "1"
print("The street is: " + street)
print("The street number is: " + street_number)
street += street_number
print("Peter is living in: " + street)
Code language: PHP (php)
Output:
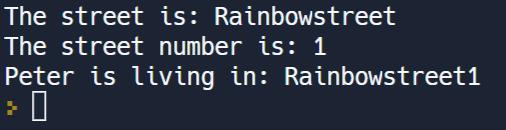
As you can see, we have the street
variable and the street_number
variable. Both values can be printed out independently. If we want to concatenate both strings together using Python str append, we can simply use the +=
operator to do that.
Python Append String Multiple Times
You can also utilize a function to append strings multiple times in Python:
multi = "Nyan"
def multiple_append(string, times):
placeholder = ""
i = 0
while i < times:
placeholder += string + " "
i = i + 1
return placeholder
multiplied_string = multiple_append(multi, 5)
print(multiplied_string)
Code language: Python (python)
Output:
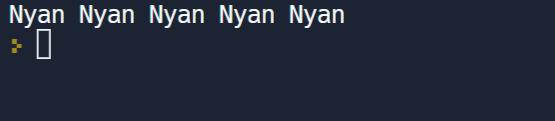
In the above example, we utilize a while
loop and our self-created multiple_append
function with its two parameters: string
and times
to concatenate the strings as many times as we defined with the times
value. The multiple_append function finally returns the string "Nyan"
five times, as defined in the multiple_append(multi, 5)
function parameter.
Python Append String using the join() Function
We can also use Python’s integrated join()
function to concatenate strings in Python. It is fairly easy to do. We first need to create a list containing all of the words we want to concatenate. Then, we use the join()
function to concatenate them all together and finally print the to the console:
first_name = "Peter"
last_name = "Parker"
temp_list = [first_name, last_name]
result = " ".join(temp_list)
print(result)
Code language: Python (python)
Output:
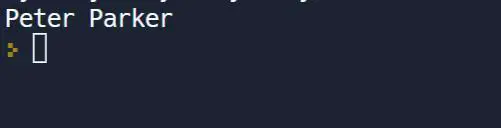
Python Append String using f-strings
F-strings are probably our favorite way of concatenating strings in Python. F-strings are really easy to use and read. Not only are they easier to write, they usually perform better than using something like the +=
operator to append strings in Python. To demonstrate how it works, check out the example below:
first_name_two = "Clark"
last_name_two = "Kent"
print(f"{first_name_two} {last_name_two}")
Code language: Python (python)
Output:
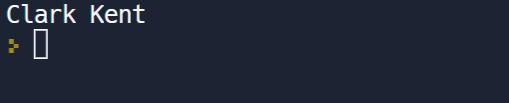
Alternatively, we can also save the concatenated string inside of a variable using the power of f-strings:
first_and_last_name = f"{first_name_two} {last_name_two}"
Code language: Python (python)
Add one Python String to Another using __add__
This is a rather exotic way of going about this, but it is possible to do it. We can utilize the __add__
method to concatenate strings in Python:
phrase_one = "Learn Python "
phrase_two = "on Ceos3c.com!"
final_phrase = phrase_one.__add__(phrase_two)
print(final_phrase)
Code language: Python (python)
Output:

This method isn’t exactly easy to read, as you can see above, so we wouldn’t recommend this one for general-purpose use in your code.
Python Str Append using the format() Function
Another way of appending strings in Python is by using yet another built-in Python function: format()
. This is another practical and useful way to concatenate strings in Python:
message_one = "Congratulations! "
message_two = "You have learned something today!"
final_message = "{}{}".format(message_one, message_two)
print(final_message)
Code language: PHP (php)
Output:

Which Method is the Best?
There isn’t really a clear answer when it comes to this question. If you just need to append one string with another in Python, using the +=
operators are perfectly fine.
If you need to concatenate more strings, using f-strings is probably a better idea. Whichever you choose, as long as you don’t extensively use string concatenation throughout your program, you should be fine with whatever method you choose!
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection