In this article, you’ll learn about Python String Length, or more specifically, how to find out the length of a string in Python. There are a couple of ways to go about this. We cover the easiest way first and also show you some alternatives that you can use to find out the length of a string in Python. This also works with variables that hold string values.
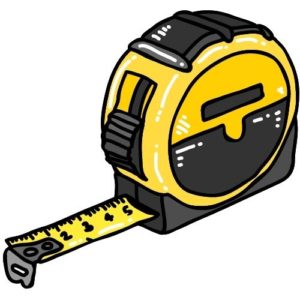
Table of Contents
Find out String Length with the len() Function
The easiest way to achieve our goal is by utilizing the built-in len()
function. To use the len()
function, we simply need to wrap the string inside the parenthesis of the len()
function.
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Basic Python len() Example
The best way to demonstrate this is by measuring the length of a string. In this case, we measure the length of the string "Hello!"
, which, including the "!"
, should amount to 6 characters of length:
our_string = "Hello!"
print(len(our_string))
Code language: PHP (php)
Output:
6
Code language: plaintext (plaintext)
The output comes back in form of an int
with the number 6, which is the length of the our_string
variable.
Using Python len() directly on a String
We can also use the len()
function directly on a string without creating a variable first:
print(len("Hello!"))
Code language: PHP (php)
Output:
6
Code language: plaintext (plaintext)
Example using Python len() with Whitespace
When checking for String Length, each whitespace is considered a character as well. If we look at the following example:
string_with_whitespace = "Hello World!"
print(len(string_with_whitespace))
Code language: PHP (php)
Output:
12
Code language: plaintext (plaintext)
The result is 12. The actual characters in the phrase “Hello World!” are just 11, but since the whitespace is also counted as a character, the result is 12.
Using a For Loop to Calculate String Length
Another way of figuring out the length of a string in Python is by using a for loop. This for loop will iterate over each character and increase the value of the variable called counter
each time the for loop encounters a string character:
counter = 0
for char in our_string:
counter +=1
print(counter)
Code language: PHP (php)
Output:
6
Code language: plaintext (plaintext)
This gives us the same result as the len()
function, which does the same without the need to write a for loop, keeping our code nice and clean.
Summary
This should give you a pretty good idea of how the Python len()
function works and also what other ways you can use to figure out the length of a string in Python.
To summarize:
- The Python
len()
function can be used to figure out the length of a string. - Whitespaces are counted as characters when using the
len()
function or a for loop. - A for loop can be used alternatively to figure out the length of a string in Python.
- The return value of the
len()
function is always an integer (number). - The
len()
function can also be used to get the length of lists, tuples, dictionaries, and other values.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection