In this blog post, we’ll be discussing how to round 2 digits after comma in Python. We’ll go over the different methods you can use to achieve this and provide a few examples for each method. Rounding numbers is a common task in programming, so it’s essential to know how to do it efficiently. With that said, let’s get started!
Table of Contents
- How do you round to 2 decimal places in Python?
- How do you round up 2 decimals in Python?
- How do you round down 2 decimals in Python?
- Rounding with string formatting in Python
- Summary
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
How do you round to 2 decimal places in Python?
There are a few different ways to round numbers in Python. The first method we’ll discuss is the built-in round()
function. This function takes two arguments: the number you want to round and the number of decimal places you want to round it to. For example, if we wanted to round the number 4.738 to two decimal places, we would write:
round(4.738, 2)
Code language: CSS (css)
Output:

This is the easiest, most straightforward way to round 2 decimals with Floats in Python. However, there are other scenarios where you might want to round up or round down.
How do you round up 2 decimals in Python?
To round up 2 decimals in Python, we can utilize the built-in math.ceil()
function, which is part of the standard math
library in Python. Let’s take the number 1.344 for this example. If we would use the round() function to round up this number:
round(1.344, 2)
Code language: CSS (css)
The output would be 1.34. This happens because the round() function follows mathematical rules and rounds everything below 5 down and everything above 5, including 5, up.
If we want to round up no matter what the number is, we first need to import the ceil()
function from the math
library, and then we can utilize ceil()
:
from math import ceil
ceil(1.344*100) / 100
Code language: JavaScript (javascript)
First, we multiply by 100 so that our number has 2 digits after the decimal point, and then we divide by 100 at the end to get back our original number.
Output:

As you can see, the number was successfully rounded up to 1.35.
How do you round down 2 decimals in Python?
Here, we can use the math
library again. This time, instead of using the ceil() function from the math library, we use the floor()
function. In this example, we try to round down the number 1.346 2 decimals, so the result should be 1.34.
First, we need to import the floor()
function from the math
library and then use the floor()
function same as we did in the example with ceil()
above:
from math import floor
print(floor(1.346 * 100) / 100)
Code language: JavaScript (javascript)
Output:
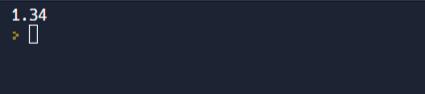
As you can see, once you understand the concept of floor()
and ceil()
, rounding up or down is really easy.
Rounding with string formatting in Python
Finally, another way to round numbers is by using string formatting with the modulo %
operator. This is our personal favorite method because it’s quick and easy once you get used to it. We also think it’s more readable than some of the other methods, but that’s just our personal opinion.
Anyway, here’s how it works: Let’s say we have the following code:
num1 = 4.738
num2 = 4.735
print("num1 rounded: " + str(round(num1 , 2)))
print("num2 rounded: " + str(round(num2 , 2)))
Code language: PHP (php)
Output:
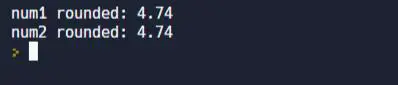
As you can see, this prints out our rounded numbers just like before. But notice that we had to convert our numbers into strings using str() before concatenating them with our other string. This is because you can’t concatenate strings with integers directly. They have to be converted into strings first.
Now, let’s take a look at how we could have done this using string formatting:
print("num1 rounded: "+ "%.2f" % num1)
print("num2 rounded: "+ "%.2f" % num2)
Code language: PHP (php)
Output:

As you can see, this code accomplishes exactly the same thing as our previous code snippet. However, we find it much easier on the eyes (and keyboard !) since we don’t have to convert our numbers into strings manually every time we want to round them.
Python string formatting edge cases
There are also some edge cases where you want to round to 2 decimal places, but the round() function will cut the second decimal point regardless due to its design. Let’s take this example:
edge_case = (150 / 5) * 1.12
edge_case_rounded = round(edge_case, 2)
print(edge_case_rounded)
Code language: PHP (php)
The result of the calculation of (150 / 5) * 1.12
is equal to 33.6. After calculating this, we throw it into our round()
function, hoping that we get 33.60 as the final result, but the final result is still 33.6.
There is a way around this issue by using string formatting. If we add another line of code to our existing code:
edge_case = (150 / 5) * 1.12
edge_case_rounded = round(edge_case, 2)
print(edge_case_rounded)
print("{:.2f}".format(edge_case_rounded))
Code language: PHP (php)
We will then get the correct output of 33.60:

Summary
As you can see, there are many different ways to use round two digits after the comma in Python, depending on your needs and preferences; these are just a few of the most popular methods among programmers today. A great way to practice rounding in Python is by trying some of our Python Exercises. In particular, Exercise 4 can be very helpful in better understanding string formatting.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection