In this tutorial, we will show you some ways to replace items in a list using Python. This is a beginner-friendly tutorial, and we will start with an easy Python List Replace method that we like.
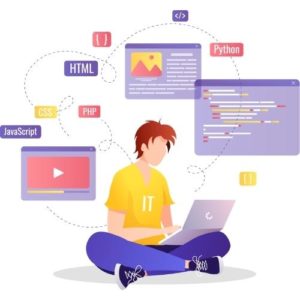
Table of Contents
- How do you replace something in a Python List?
- Examples
- Method 1 – Python List Replace using Index
- Method 2 – Python Replace Element in List using Python enumerate()
- Method 3 – For Loop
- Method 4 – While Loop
- Method 5 – Lambda Function with map()
- Method 6 – List Replace using List Slicing
- Summary Python List Replace
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
How do you replace something in a Python List?
To replace something in a Python list, we can use:
- List Indexing
- For Loops
- The
enumerate()
function - While Loops
- Lambda Functions with
map()
- List Slicing
Examples
Let’s assume we have a list of different items, and we want to replace an element of this list with a specific index.
Our list:
favorite_colors = ["Red", "Gray", "Blue", "Yellow", "Magenta", "Brown"]
Code language: JavaScript (javascript)
As everything changes, so does our taste for colors. We just don’t like Red anymore, and we decide to replace it with something more substantial and classy – Black. But how do we do that? How do we replace an element in a list in Python?
We’ll show you a couple of different ways below, ultimately replacing every single color of our original list using different methods.
Method 1 – Python List Replace using Index
Probably the easiest way to replace an element in a list in Python is by re-assigning the value at a specific index. To do this with our favorite_colors
list, we can simply use the index of “Red” (0) and replace it with "Black"
.
favorite_colors[0] = "Black"
print(favorite_colors)
Code language: Python (python)
Output
['Black', 'Gray', 'Blue', 'Yellow', 'Magenta', 'Brown']
Code language: Bash (bash)
That is a pretty simple and easy-to-understand way of replacing a list item in Python.
Method 2 – Python Replace Element in List using Python enumerate()
Another way of achieving our goal would be to use enumeration with the enumerate()
function:
for index, color in enumerate(favorite_colors):
if color == "Gray":
favorite_colors[index] = "Beige"
print(favorite_colors)
Code language: Python (python)
Output:
['Black', 'Beige', 'Blue', 'Yellow', 'Magenta', 'Brown']
Code language: plaintext (plaintext)
This way, we utilize a for loop in combination with the enumerate()
function to locate the color "Gray"
and replace it with the color "Beige"
.
Breaking this down, the enumerate()
function requires three things:
- A placeholder for the index
- A placeholder for the item (in our case, the color)
- And the enumerate() function with our list in its parenthesis
The loop then runs over each item of our list and checks if the color is, in fact, "Gray"
. If that is the case, we use the same principle as in Method 1 to replace that item at the current index with our new color "Beige"
. The current index of the color "Gray"
is here represented by favorite_colors[index]
.
That is essentially the same as favorite_colors[1]
as in Method 1 above, but that way, we don’t need to manually count the index of the color we want to replace. We can use logic and if/else statements to do that for us.
Method 3 – For Loop
We can also use a For Loop to loop over our list and replace an item that matches our condition:
for i in range(len(favorite_colors)):
# Replace "Red" with "Purple"
if favorite_colors[i] == 'Blue':
favorite_colors[i] = 'Purple'
print(favorite_colors)
Code language: Python (python)
Output:
['Black', 'Beige', 'Purple', 'Yellow', 'Magenta', 'Brown']
Code language: plaintext (plaintext)
Method 4 – While Loop
While we can use a While Loop to replace list items, we wouldn’t recommend it:
i = 0
while i < len(favorite_colors):
# Replacing "Blue" with "Green"
if favorite_colors[i] == "Yellow":
favorite_colors[i] = "Green"
i += 1
print(favorite_colors)
Code language: Python (python)
Output:
['Black', 'Beige', 'Purple', 'Green', 'Magenta', 'Brown']
Code language: plaintext (plaintext)
Method 5 – Lambda Function with map()
We can use the anonymous lambda function in combination with the map()
function to iterate over our list and replace an item. Once the iteration is done, we use the list()
function to convert the temporary map into a list:
# List Replace using Lambda Function
favorite_colors = list(map(lambda x: x.replace('Magenta', "Cyan"), favorite_colors))
print(favorite_colors)
Code language: Python (python)
Output:
['Black', 'Beige', 'Purple', 'Green', 'Cyan', 'Brown']
Code language: plaintext (plaintext)
Method 6 – List Replace using List Slicing
List slicing is another great way to replace list items in Python. This is how it works:
- Finding the item we want to replace and store it in the variable
idx
- Replacing the item with the item stored inside of
idx
with the item in the list using list slicing syntax
This can be a bit hard to understand, so here is the syntax:
favorite_colors=favorite_colors[:index]+["Violet"] + [index+1:]
Code language: Python (python)
Here is an example:
# List Replace using List Slicing
idx = favorite_colors.index("Brown")
favorite_colors = favorite_colors[:i] + ["Violet"] + favorite_colors[i+1:]
print(favorite_colors)
Code language: Python (python)
Output:
['Black', 'Beige', 'Purple', 'Green', 'Cyan', 'Brown', 'Violet']
Code language: plaintext (plaintext)
Summary Python List Replace
When it comes to pure replacements, those are the most common methods you will come across when you want to replace elements in a list in Python. There are also things like Python List Comprehensions, but that kind of defeats the purpose of replacing items.
Another way to work with lists in Python is by filtering them. Depending on your use case, one of the two functions should fit your needs. If you want to learn how to add items to lists in Python, check out this article.
To summarize:
- We can replace items using List Indexing
- We can replace items using the
enumerate()
function - We can replace items using For Loops
- We can replace items using While Loops
- We can replace items using a Lambda Function with
map()
- We can replace items using List Slicing
If you want to check out some other programming tutorials, have a look at some related articles.
🐍 Learn Python Programming
🔨 Python Basics
👉 Python Syntax
👉 Python Variables
👉 Python print()
👉 Python input()
👉 Python Constants
👉 Python Comments
⚙️ Python Specifics
👉 Filter Lists in Python
👉 Replacing List Items in Python
👉 Create a GUI in Python
👉 Find out the Length of a String in Python
👉 Enum in Python
👉 Python Inline If/Else One-Line Statements
👉 Python xrange()
👉 Python List Slicing
🏋️ Python Exercises
👉 Python Exercise Collection
Very understandably explained! Thanks!
Thanks, Lubos!