Sorting numbers in JavaScript is a fundamental task for web developers. There are several ways to sort numbers in JavaScript, ranging from basic to advanced methods. In this article, we’ll explore everything there is to know about sorting numbers in JavaScript, from beginner to advanced techniques, with lots of examples.
Table of Contents
- Sort Numbers in JavaScript using an Array
- Basic Sorting using Array.sort()
- How the sort() function works
- Sorting Negative Numbers
- Sorting an Array of Mixed Numbers and Strings
- Sorting Arrays of Objects
- Advanced Sorting Algorithms
- Conclusion
ℹ️ This article has a corresponding Replit repository that you can copy and play around with. Create a free Replit account and click on the Fork Repl button below. This is the best way to practice programming!
Sort Numbers in JavaScript using an Array
Alright, let’s dive right into the code:
// Our Numbers Array
let numbers = [22, 50, 1, 2, 39, 29, 10]
// Our sort function
function sortNumbers(array) {
array.sort((a, b) => a - b)
}
// Call the function
sortNumbers(numbers)
// Log the result
console.log(numbers)
// How the function works
function compareNumbers(a, b) {
console.log(a - b)
}
compareNumbers(1, 5)
Code language: JavaScript (javascript)
This is as simple as it gets. JavaScript provides us with the sort()
function, so we can utilize that. Let’s break the code down.
- We declare our array of numbers.
- We create a function that takes our array as an argument and then runs the sort function on the array.
- We are calling our
sortNumbers(numbers)
function with our numbers array as an argument. - We
console.log
our now sorted numbers array.
The output looks as follows:
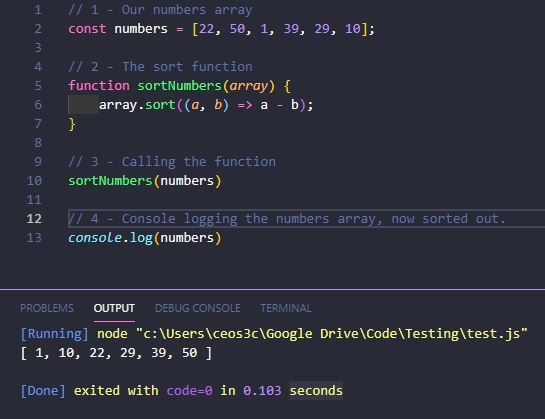
Basic Sorting using Array.sort()
The most basic way to sort numbers in JavaScript is by using the Array.sort()
method. This method sorts the elements of an array in place and returns the sorted array. By default, the Array.sort()
method sorts the elements in ascending order.
const numbers = [5, 2, 1, 4, 3];
numbers.sort(); // [1, 2, 3, 4, 5]
Code language: JavaScript (javascript)
To sort numbers in descending order, we can use a compare function as a parameter to Array.sort()
. The compare function should return a negative value if the first argument is less than the second argument, a positive value if the first argument is greater than the second argument, and 0 if they are equal.
const numbers = [5, 2, 1, 4, 3];
numbers.sort((a, b) => b - a); // [5, 4, 3, 2, 1]
Code language: JavaScript (javascript)
How the sort() function works
The probably hardest part to understand in this code is how the actual sort()
function works. Let’s break it down then, shall we?
The version I used in my code is simply a shorter version of the original function that was introduced in ES2015.
array.sort((a, b) => a - b)
Code language: JavaScript (javascript)
Let us look at the original version, which is maybe a bit easier to understand:
array.sort(function(a, b) {
return a - b;
});
Code language: JavaScript (javascript)
In essence, the function subtracts b from a, and if a positive value is returned, a is larger than b. If 0 is returned, both values are equal, and if something >0 is returned, a is less than b.
sort(1, 5) // It returns -4, meaning a is less than b.
Code language: JavaScript (javascript)
If you want to dig deeper into the ins and outs of this function, there is a great Stackoverflow answer to it. You can read it here.
Sorting Negative Numbers
When sorting an array of negative numbers, we need to use a slightly different compare function to ensure that the sort order is correct.
const numbers = [-5, -2, -1, -4, -3];
numbers.sort((a, b) => a - b); // [-5, -4, -3, -2, -1]
numbers.sort((a, b) => b - a); // [-1, -2, -3, -4, -5]
Code language: JavaScript (javascript)
Sorting an Array of Mixed Numbers and Strings
When sorting an array of mixed numbers and strings, the Array.sort()
method may not work as expected. To sort an array of mixed numbers and strings, we can use a compare function that converts the elements to a common data type before comparing them.
const mixed = ['10', 5, '3', -1, '2'];
mixed.sort((a, b) => a - b); // [-1, '2', '3', 5, '10']
mixed.sort((a, b) => Number(a) - Number(b)); // [-1, 2, 3, 5, 10]
Code language: JavaScript (javascript)
Sorting Arrays of Objects
When sorting arrays of objects, we need to use a compare function that compares a specific property of the objects.
const people = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 20 },
];
people.sort((a, b) => a.age - b.age); // [{ name: 'Charlie', age: 20 }, { name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }]
Code language: JavaScript (javascript)
Advanced Sorting Algorithms
While the Array.sort()
method is sufficient for most sorting tasks, more advanced sorting algorithms may be required for certain scenarios.
Merge Sort
Merge sort is a divide-and-conquer algorithm that divides the input array into smaller sub-arrays, sorts the sub-arrays, and merges them back together.
function mergeSort(arr) {
if (arr.length <= 1) {
return arr;
}
const middle = Math.floor(arr.length / 2);
const left = arr.slice(0, middle);
const right = arr.slice(middle);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let i = 0;
let j = 0;
while (i < left.length && j < right.length) {
if (left[i] < right[j]) {
result.push(left[i]);
i++;
} else {
result.push(right[j]);
j++;
}
}
return result.concat(left.slice(i)).concat(right.slice(j));
}
const numbers = [5, 2, 1, 4, 3];
mergeSort(numbers); // [1, 2, 3, 4, 5]
Code language: JavaScript (javascript)
Quick Sort
Quick sort is another divide-and-conquer algorithm that selects a pivot element and partitions the array into two sub-arrays, one with elements less than the pivot and one with elements greater than the pivot.
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[Math.floor(Math.random() * arr.length)];
const left = [];
const right = [];
for (let i = 0; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else if (arr[i] > pivot) {
right.push(arr[i]);
}
}
return quickSort(left).concat(pivot, quickSort(right));
}
const numbers = [5, 2, 1, 4, 3];
quickSort(numbers); // [1, 2, 3, 4, 5]
Code language: JavaScript (javascript)
Heap Sort
Heap sort is a comparison-based sorting algorithm that uses a binary heap data structure to sort the elements. Heap sort has a time complexity of O(n log n).
function heapSort(arr) {
buildHeap(arr);
for (let i = arr.length - 1; i > 0; i--) {
swap(arr, 0, i);
heapify(arr, 0, i);
}
return arr;
}
function buildHeap(arr) {
const length = arr.length;
for (let i = Math.floor(length / 2); i >= 0; i--) {
heapify(arr, i, length);
}
}
function heapify(arr, i, length) {
const left = 2 * i + 1;
const right = 2 * i + 2;
let max = i;
if (left < length && arr[left] > arr[max]) {
max = left;
}
if (right < length && arr[right] > arr[max]) {
max = right;
}
if (max !== i) {
swap(arr, i, max);
heapify(arr, max, length);
}
}
function swap(arr, i, j) {
const temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
const numbers = [5, 2, 1, 4, 3];
heapSort(numbers); // [1, 2, 3, 4, 5]
Code language: JavaScript (javascript)
Conclusion
In conclusion, there are several ways to sort numbers in JavaScript, from basic to advanced techniques. The most basic method is using the Array.sort()
method, which can sort an array of numbers in ascending or descending order. To sort arrays of mixed numbers and strings or arrays of objects, we can use a compare function that converts the elements to a common data type or compares a specific property of the objects.
For more advanced sorting scenarios, we can use more advanced sorting algorithms such as merge sort, quick sort, or heap sort. These algorithms have better time complexity than the Array.sort()
method and are more suitable for sorting large arrays or complex data structures.
It’s important to note that while the advanced sorting algorithms may have better time complexity, they may also have higher memory usage or require more code to implement. It’s always important to consider the trade-offs between time complexity, memory usage, and code complexity when choosing a sorting algorithm for a particular scenario.
Overall, understanding how to sort numbers in JavaScript is an important skill for web developers. By using the different sorting techniques and algorithms discussed in this article, developers can ensure that their applications can efficiently sort arrays of numbers and other data structures, leading to better performance and user experience.
If you want to learn how to find out if a certain value is present in an array, you might want to read up on the JavaScript includes() method!
Awesome my friend. Why you delete ezoic review article?
I did not. You can find it here: https://www.ceos3c.com/reviews/ezoic-review-how-ezoic-helped-me-to-go-full-time-blogger/
Thank you