Working with arrays is a fundamental aspect of JavaScript programming, and there are several ways to iterate through the elements of an array. One of the most popular methods for iterating over an array in JavaScript is the forEach()
method. In this article, we will explore the JavaScript forEach() method in detail and provide some clear and concise examples to help you understand how it works.
Whether you are a beginner or an experienced developer, understanding how to work with arrays is crucial to building robust JavaScript applications. As you learn about arrays, you will inevitably come across the forEach()
method, which is a powerful tool for iterating over arrays and executing a predefined function on each element.
In this article, we will cover the basics of forEach()
and provide some simple examples that will help you understand how it works. Whether you are new to programming or looking to expand your knowledge of JavaScript, this article will provide you with the knowledge you need to start using forEach()
with confidence.
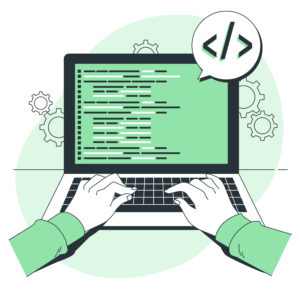
Table of Contents
- What Is the JavaScript forEach() Method?
- Why Use the JavaScript forEach() Method?
- Understanding the JavaScript forEach() Method
- Parameters of the JavaScript forEach() Method
- Comparison between for() loop and forEach() loop
- More Examples Using the forEach() Loop
- Conclusion
What Is the JavaScript forEach() Method?
The for()
loop is a classic way of iterating over arrays, but it has some limitations. The forEach()
method in JavaScript is a more powerful and flexible way of iterating over arrays, as it allows you to execute a callback function for each element of an array.
Here’s the basic syntax for using the forEach()
method:
array.forEach(function(currentValue, index, arr) {
// Your code here
});
Code language: PHP (php)
The forEach()
method takes a function as its argument, which is called for each element of the array. The function takes three arguments:
currentValue
: The value of the current element being processedindex
(optional): The index of the current element being processedarr
(optional): The array being traversed
The index
and arr
parameters are optional. The index
parameter specifies the index of the current element being processed, and the arr
parameter specifies the array being traversed.
The forEach()
method is a concise and expressive way of iterating over arrays in JavaScript. Here’s an example of using forEach()
to iterate over an array of numbers and log each one to the console:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
Code language: JavaScript (javascript)
In this example, we create an array of numbers and use the forEach()
method to loop over each element of the array. The function passed to forEach()
takes a single parameter, number
, which represents the current element being processed. We then log the current number
to the console.
The return value of the forEach()
method is always undefined
. This means that the forEach()
method does not return anything, but simply executes the callback function for each element of the array.
In summary, the forEach()
method is a powerful and flexible way of iterating over arrays in JavaScript. It allows you to execute a callback function for each element of an array, making it a concise and expressive way of working with arrays.
Why Use the JavaScript forEach() Method?
When working with arrays in JavaScript, it’s common to need to iterate over the elements of the array and perform some operation on each element. There are several ways to do this in JavaScript, but one of the most powerful and flexible ways is to use the forEach()
method.
The forEach()
method allows you to loop over the elements of an array and execute a callback function for each element. This makes it easy to perform operations on each element of an array without the need for a complex loop structure.
Here’s an example of using the forEach()
method to loop over an array of numbers and log each one to the console:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
Code language: JavaScript (javascript)
In this example, we use the forEach()
method to loop over an array of numbers and execute a callback function for each number. The callback function takes a single argument, number
, which represents the current element being processed. We then log the current number
to the console.
Using the forEach()
method can make your code more readable and easier to maintain. It eliminates the need for complex loop structures and allows you to focus on the logic of your code rather than the mechanics of iterating over an array.
In addition to being easy to use, the forEach()
method is also very flexible. You can pass in an arrow function or a named function as the callback function, and you can also access the index and array parameters if needed.
Understanding the JavaScript forEach() Method
The forEach()
method in JavaScript is a powerful tool for iterating over arrays, sets, and maps, and executing a callback function for each element. The forEach()
method takes a callback function as its argument, which is executed once for each element in the array or collection.
Here’s the basic syntax for using the forEach()
method:
array.forEach(function(item, index, arr) {
// Your code here
});
Code language: PHP (php)
The forEach()
method takes a function as its argument, which is called for each element of the array or collection. The function takes three arguments:
item
: The value of the current element being processedindex
(optional): The index of the current element being processedarr
(optional): The array or collection being traversed
The index
and arr
parameters are optional. The index
parameter specifies the index of the current element being processed, and the arr
parameter specifies the array or collection being traversed.
Here’s an example of using the forEach()
method to loop over an array of numbers and log each one to the console:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
Code language: JavaScript (javascript)
In this example, we use the forEach()
method to loop over an array of numbers and execute a callback function for each number. The callback function takes a single argument, number
, which represents the current element being processed. We then log the current number
to the console.
You can also access the index and array parameters if needed. Here’s an example of using the index parameter to log the index of each element in the array:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number, index) {
console.log(`Number ${number} has index ${index}`);
});
Code language: JavaScript (javascript)
In this example, we use the index
parameter to log the index of each element in the array.
You can also use arrow functions with the forEach()
method, which can make your code more concise and easier to read. Here’s an example of using an arrow function to log each element in an array:
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(fruit => console.log(fruit));
Code language: JavaScript (javascript)
In this example, we use an arrow function to log each element in an array of fruits.
Parameters of the JavaScript forEach() Method
The forEach()
method in JavaScript takes two optional parameters and two optional arguments that can be passed to the callback function.
1. Callback Function
The callback function is the function that performs the required action on each element of the array during the iteration. The forEach()
method executes the callback function once for each element of the array, passing the element, index, and array as arguments.
Here’s an example of using a callback function with the forEach()
method:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
Code language: JavaScript (javascript)
In this example, we use a callback function to log each element of the numbers
array to the console.
2. thisArg
The thisArg
parameter is an optional value that can be used as the this
value when executing the callback function. If this argument is provided, the callback function is called with this
set to the value of thisArg
.
Here’s an example of using the thisArg
parameter with the forEach()
method:
function Counter() {
this.sum = 0;
this.count = 0;
}
Counter.prototype.add = function(array) {
array.forEach(function countEntry(entry) {
this.sum += entry;
++this.count;
}, this);
};
const obj = new Counter();
obj.add([8, 7, 12]);
console.log(obj.count); // Output: 3
console.log(obj.sum); // Output: 27
Code language: JavaScript (javascript)
In this example, we use the thisArg
parameter to set the value of this
inside the callback function to the Counter
object.
3. Index
The index
parameter refers to the index value of the current element being processed during the iteration. This parameter is optional and is passed to the callback function as the second argument.
Here’s an example of using the index
parameter with the forEach()
method:
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(function(fruit, index) {
console.log(`Fruit ${fruit} has index ${index}`);
});
Code language: JavaScript (javascript)
In this example, we use the index
parameter to log the index of each element in the fruits
array.
4. Array
The array
parameter refers to the array being traversed by the forEach()
method. This parameter is optional and is passed to the callback function as the third argument.
Here’s an example of using the array
parameter with the forEach()
method:
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(function(fruit, index, array) {
console.log(`Fruit ${fruit} has index ${index} in array ${array}`);
});
Code language: JavaScript (javascript)
In this example, we use the array
parameter to log the array being traversed by the forEach()
method.
Comparison between for() loop and forEach() loop
Both the for()
loop and the forEach()
loop are used to execute a set of statements multiple times, but their functionalities differ.
The for()
loop:
- Is one of the most common iteration methods used to execute code several times.
- Is faster when it comes to executing statements, making it suitable for time-sensitive operations.
- Can be harder to read and write, especially for beginners.
- Is a general-purpose loop that can be used with various data structures, including arrays, objects, and maps.
Here’s an example of using a for()
loop to iterate over an array of numbers and log each number to the console:
const numbers = [1, 2, 3, 4, 5];
for(let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
Code language: JavaScript (javascript)
In this example, we use a for()
loop to log each number of the numbers
array to the console.
The forEach()
loop:
- Is a newer iteration method used to iterate over the elements of an array.
- Can be slower in terms of traversing and executing statements, making it less suitable for time-sensitive operations.
- Is easier to read and write than the
for()
loop, making it more beginner-friendly. - Is specifically designed to work with arrays, making it a more specialized loop.
Here’s an example of using a forEach()
loop to iterate over an array of fruits and log each fruit to the console:
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(function(fruit) {
console.log(fruit);
});
Code language: JavaScript (javascript)
In this example, we use a forEach()
loop to log each fruit of the fruits
array to the console.
In summary, both the for()
loop and the forEach()
loop have their strengths and weaknesses, and the choice of which to use depends on the specific requirements of the task at hand. However, in general, the for()
loop is more versatile, while the forEach()
loop is more specialized and beginner-friendly.
More Examples Using the forEach() Loop
The forEach()
loop is a great tool for iterating over arrays and performing operations on their elements. Here are some examples that demonstrate the power and flexibility of this loop:
Example 1: Summing the Values of an Array
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
numbers.forEach(function(number) {
sum += number;
});
console.log(sum); // Output: 15
Code language: JavaScript (javascript)
In this example, we use the forEach()
loop to sum the values of an array of numbers. We initialize a sum
variable to zero and then add each number in the array to it using the callback function.
Example 2: Filtering an Array
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = [];
numbers.forEach(function(number) {
if(number % 2 === 0) {
evenNumbers.push(number);
}
});
console.log(evenNumbers); // Output: [2, 4]
Code language: JavaScript (javascript)
In this example, we use the forEach()
loop to filter an array of numbers and create a new array containing only the even numbers. We initialize an empty evenNumbers
array and then check each number in the array using the callback function. If the number is even, we push it into the evenNumbers
array.
Example 3: Manipulating the Elements of an Array
const names = ['John', 'Jane', 'Michael'];
const newNames = [];
names.forEach(function(name) {
const newName = name.toUpperCase();
newNames.push(newName);
});
console.log(newNames); // Output: ["JOHN", "JANE", "MICHAEL"]
Code language: JavaScript (javascript)
In this example, we use the forEach()
loop to manipulate the elements of an array of names. We create a new array called newNames
and then use the callback function to convert each name to uppercase using the toUpperCase()
method and push the new name into the newNames
array.
Example 4: Updating the Properties of an Object
const people = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Michael', age: 35 }
];
people.forEach(function(person) {
person.age += 1;
});
console.log(people);
/*
Output:
[
{ name: 'John', age: 26 },
{ name: 'Jane', age: 31 },
{ name: 'Michael', age: 36 }
]
*/
Code language: JavaScript (javascript)
In this example, we use the forEach()
loop to update the properties of objects in an array. We have an array of people objects with a name
and an age
property. We use the callback function to add 1 to the age
property of each object in the array.
Conclusion
In this article, we have covered the JavaScript forEach() loop, explaining its syntax, parameters, and return value. We have also provided several examples that demonstrate the power and versatility of this loop, including summing the values of an array, filtering an array, manipulating the elements of an array, and updating the properties of an object.
If you want to learn more about JavaScript and web development, we recommend checking out the following articles on ceos3c.com:
- The JavaScript Array find() Method Made Easy: An in-depth explanation of the find() method for arrays in JavaScript.
- JavaScript Slice Arrays: Simplified: An overview of the slice() method for arrays in JavaScript.
With enough practice and exploration, you’ll become a master of the forEach() loop and be able to use it to its full potential in your JavaScript code.