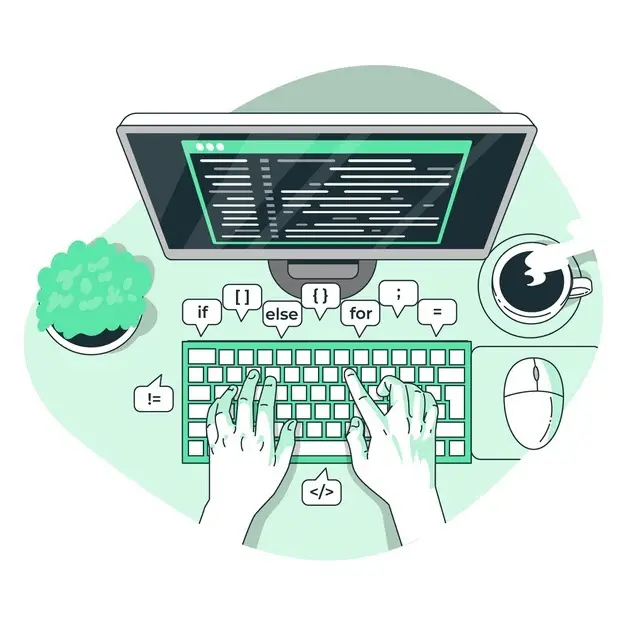
Have you ever come across a coding term or attribute and wondered what it meant? Maybe you know the definition, but you’re having difficulty comprehending the term. We’ve got you. Not only you, but generally every beginner struggles to decipher technical jargon and definitions. So, let us help you understand the JavaScript Slice or JS Slice method in JavaScript (for arrays).
You’ll find the JavaScript Slice for strings version here.
🔥 Learn JavaScript Programming – Beginner Friendly!
🔨 JavaScript Basics
👉 JavaScript alert()
👉 Difference between Let and Const
🔸 JavaScript Arrays
👉 JavaScript Array Filter
👉 JavaScript Array Find
👉 JavaScript forEach (Arrays)
👉 JavaScript Slice (Arrays)
👉 JavaScript Spread Operator
🔸 JavaScript Strings
👉 JavaScript Slice(Strings)
👉 JavaScript Includes
🌐 Web Development with JavaScript
👉 Store User Input in a variable with JS
⚙️ JavaScript Specifics
👉 Sorting Numbers in JS
👉 JavaScript Fetch API
👉 toLocaleDateString in JavaScript
Table of Contents
- What Is the JavaScript slice() Method?
- The Syntax of JS slice()
- Practical Examples
- JavaScript Slice() Method Example
- Converting Array-Like Objects Into Arrays
- Browsers That Support JavaScript slice() Method
- Wrapping Up
What Is the JavaScript slice() Method?
Let’s say you are creating an array of fruits. You name the array ‘fruits‘ and add some fruit names into the array.
Now you want to create two more arrays and name them ‘Tropical fruits‘ and ‘Citrus fruits‘. You will do the same, create two arrays and add the fruit names that belong to that category.
This is when the slice()
method comes in handy. Instead of repeatedly adding contents to different arrays, the slice()
method allows you to select and add elements from another existing array to the new array.
👀 Learn JavaScript the Easy Way (🦄 ADHD Friendly!)
👉 JavaScript Array Filter – Made Easy
👉 JavaScript Array Find – Made Easy
👉 JavaScript forEach – Made Easy
👉 Difference between Let and Const
👉 Sorting Numbers in JS!
👉 Store User Input in a variable with JS!
👉 JavaScript Spread Operator – Made Easy
👉 JavaScript Slice (Arrays) – Made Easy
👉 JavaScript Slice(Strings) – Made Easy
👉 JavaScript Includes – Made Easy
The Syntax of JS slice()
array.slice(start, end)
In which,
- The array refers to the array’s name from which we will be getting the elements.
- The start refers to the starting index from which the elements will be extracted. By default, the starting index is 0.
- The end refers to the last index until which the elements will be extracted. In default, the last position is the last element of the array.
The Return Value
The new array containing the requested elements taken from another array will be returned as the return value.
Practical Examples
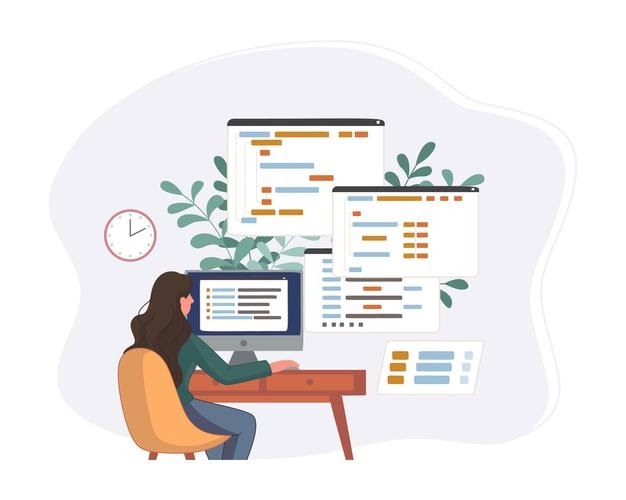
It is essential to look into practical examples to comprehend programming concepts fully. Let’s look into the examples demonstrating the implementation of the slice()
method.
Example 1
In this example, we will not be utilizing any arguments. So, the slice()
method copies all the elements into the new array.
let fruits = ["Banana","Apple","Orange","Mango","Citrus"];
let new_arr = fruits.slice();
console.log(fruits);
console.log(new_arr);
Code language: JavaScript (javascript)
Output:
[Banana,Apple,Orange,Mango,Citrus]
[Banana,Apple,Orange,Mango,Citrus]
Code language: Bash (bash)
Example 2
In this example, we will use one argument, ‘2’. This means elements starting from position 2 till the last element will be copied.
let fruits = ["Banana","Apple","Orange","Mango","Citrus"];
let new_arr = fruits.slice(2);
console.log(fruits);
console.log(new_arr);
Code language: JavaScript (javascript)
Output:
[Banana, Apple, Orange, Mango, Citrus]
[Orange, Mango, Citrus]
Code language: Bash (bash)
Example 3
We will create a new array in this example, and extract the tropical fruits from the main array, fruits, to the new array, tropical.
let fruits = ["Banana","Mango","Apple","Orange","Citrus"];
let tropical = fruits.slice(0,2);
console.log(fruits);
console.log(tropical);
Code language: JavaScript (javascript)
Output:
[Banana,Mango,Apple,Orange,Citrus]
[Banana,Mango]
Code language: JSON / JSON with Comments (json)
JavaScript Slice() Method Example
Drop this code below inside of a repl.it (Select the HTML, CSS, JS template and drop it inside the .js file) and run it.
function func() {
// Original Array
let fruits = ["Banana","Mango","Apple","Orange","Lime"];
// Extracted Array
let tropical = fruits.slice(0,2);
let citrus = fruits.slice(3,5);
document.write(fruits);
document.write("<br>");
document.write(tropical);
document.write("<br>");
document.write(citrus);
}
func();
Code language: JavaScript (javascript)
Output:
[Banana,Mango,Apple,Orange,Lime]
[Banana,Mango]
[Orange,Lime]
Code language: JSON / JSON with Comments (json)
Converting Array-Like Objects Into Arrays
Till now, we saw examples of the slice()
method slicing the elements of an array into different arrays. Not only does the slice()
method convert arrays into newer arrays, but it also helps in converting array-like objects into arrays too.
To understand it clearly,
Example:
function newArray() {
return Array.prototype.slice.call(arguments);
}
let classification = newArray('C','D','E');
console.log(classification); // ["C", "D", "E"]
Code language: JavaScript (javascript)
In the above example, the newArray()
function has a series of arguments that looks the same as the elements in an array, which is why we refer to them as array-like objects. So, we are using the slice()
method to convert these array-like objects into actual arrays.
Hence, every argument we add in the newArray()
function will become the new array element.
Browsers That Support JavaScript slice() Method
The JS slice ()
method is compatible with any browser since it is an ECMAScript1 (ES1) feature. The browsers that support the slice()
method are:
- Chrome
- Edge
- Firefox
- Safari
- Opera
Wrapping Up
We hope you now know what the slice()
method is, how to use it in your code, and what the different sorts of implementations are. It is vital to learn and practice coding to become a skilled programmer, so try out the concepts you learned today.