The JavaScript alert function is used to display alerts, or easier said, notifications to a user of a website. To use this function, the first job of a developer is to have a good grasp of every useful feature that JavaScript offers. Now let’s dive into this article to learn what the JavaScript alert()
function is about and how it is used through coding examples.
Table of Contents
- What Is a JavaScript alert()?
- What Is the Use of the alert() Method?
- How to Prevent an Alert Message?
- Browsers That Support JavaScript alert() Method
- JavaScript alert() and Cross-Site Scripting (XSS)
- Wrapping Up
🔥 Learn JavaScript Programming – Beginner Friendly!
🔨 JavaScript Basics
👉 JavaScript alert()
👉 Difference between Let and Const
🔸 JavaScript Arrays
👉 JavaScript Array Filter
👉 JavaScript Array Find
👉 JavaScript forEach (Arrays)
👉 JavaScript Slice (Arrays)
👉 JavaScript Spread Operator
🔸 JavaScript Strings
👉 JavaScript Slice(Strings)
👉 JavaScript Includes
🌐 Web Development with JavaScript
👉 Store User Input in a variable with JS
⚙️ JavaScript Specifics
👉 Sorting Numbers in JS
👉 JavaScript Fetch API
👉 toLocaleDateString in JavaScript
What Is a JavaScript alert()?
The JavaScript alert()
function is used to display a warning box that pops at the top of the page with a warning message with an ‘OK’ button and sometimes with a ‘Cancel’ button. The purpose of the alert()
function is to deliver the user a message and ask their permission to continue the process.
The syntax of JavaScript alert()
is as follows where the message or warning intended to display to the user is included within the brackets.
alert(message or warning);
Code language: JavaScript (javascript)
We will use the alert()
function in the following example. Here, a button is created; the purpose of this button is that when a user clicks on it, the page will display the alert box.
<!DOCTYPE html>
<html lang="en">
<body>
<div style="margin-left: 250px; margin-top: 250px;">
<button style="font-size: 20px;"
class="btn" onclick="fun()">
Click to display the Alert Box
</button>
</div>
<script>
function fun(){
alert("Here is an example of JS alert()!");
}
</script>
</body>
</html>
Code language: JavaScript (javascript)
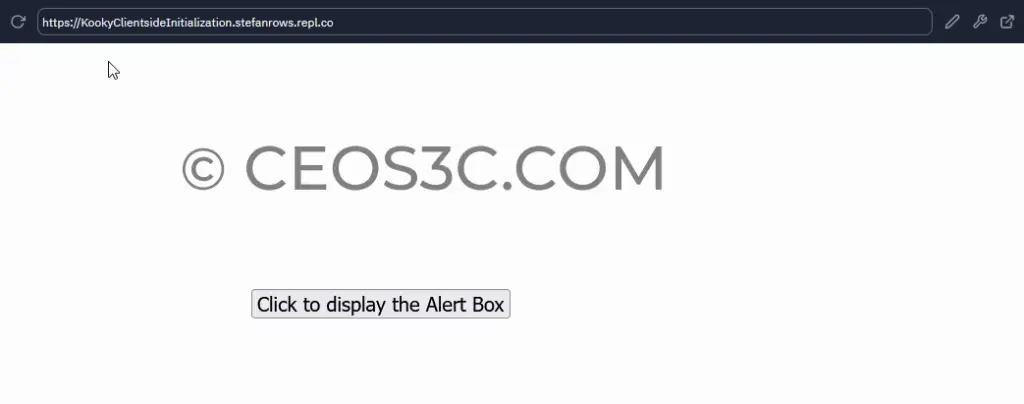
Output
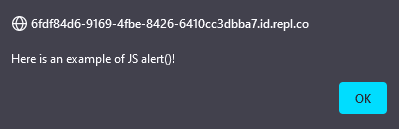
The output is the button. When clicked, the alert box with the message, “Here is an example of JS alert()!” will be presented.
What Is the Use of the alert() Method?
Imagine a user visiting a social media website. They will enter their login information and click Login on the login page. If they have entered the accurate info, they will be directed to their account page; however, if they have got something wrong, the page will display a warning box informing them that they have entered the incorrect information.
Imagine a user visiting a social media website. They will enter their login information and click Login on the login page. If they have entered the accurate info, they will be directed to their account page; however, if they have got something wrong, the page will display a warning box informing them that they have entered the incorrect information.
- The alert message is displayed when conditions are not met
A form is used in the example below to gather a user’s personal information. If the user is under 18, a warning will be displayed thanks to the alert()
function.
In HTML
<form name="myForm" >
<label for="age">Age</label>
<input type="number" name="age" id="age" />
<button type="submit">Submit</button>
</form>
Code language: HTML, XML (xml)
In JavaScript
const checkAge = (e) => {
if(document.querySelector('#age').value < 18){
// Preventing the submit of the form
e.preventDefault();
// Displaying the modal window
alert("To continue browsing, you need to be above 18");
}
};
// Listening to the click event on the button
document.querySelector('button').addEventListener('click', checkAge);
Code language: JavaScript (javascript)
That is the purpose of the alert()
method. Not only when entering incorrect details, but the alert()
method can also be used to ask the user for final permission if they are ready to perform a specific activity.
- The alert message displayed when waiting for confirmation
For example, if you are downloading an extension from a chrome web store, you will be asked whether you want to download the extension in the browser. If yes, the extension will be downloaded, and if you choose to cancel, it won’t be downloaded.
The alert()
function is used in the following example to display a confirmation message inquiring whether the user wishes to download software or not.
In HTML
<button type="submit">Download</button>
Code language: JavaScript (javascript)
In JavaScript
const showAlert = (e) => {
alert('Do you want to Download the program?');
};
document.querySelector('button').addEventListener('click', showAlert);
Code language: JavaScript (javascript)
- The alert message displayed when loading web pages
In the following example, the alert()
function represents the alert box when the page is reloaded.
<!DOCTYPE html>
<html lang="en">
<head>
<script>
alert("The alert box appears when the page is loaded!");
</script>
</head>
<body>
When you click run, you will be presented with the alert box.
</body>
</html>
Code language: HTML, XML (xml)
The above example types are frequently used in web development; for example, if we leave a page without filling a form, we will be presented with a box asking whether we intend to leave the page without completing the form.
- The alert message displayed to receive input from the user
In the following example, we will use the alert()
function to receive input from the user through the alert box.
In HTML
<button type="submit">Enter Name</button>
Code language: HTML, XML (xml)
In JavaScript
const showAlert = (e) => {
alert('Enter Your Name', 'Name');
};
document.querySelector('button').addEventListener('click', showAlert);
Code language: JavaScript (javascript)
How to Prevent an Alert Message?
Sometimes the alert()
function can be pretty annoying for the users. Though no in-built function is available on the browsers to mute this feature, users can simply create another empty alert()
function to overwrite the previous one.
window.alert = function() {};
Code language: JavaScript (javascript)
Or
alert = function() {};
Code language: JavaScript (javascript)
The changes made are only for your browser alone. Though most browsers do not provide any options for blocking alert messages, browsers such as Firefox allow users to disable the alert messages if they appear too much.
Browsers That Support JavaScript alert() Method
Every known browser supports the JavaScript
method since its role is crucial in warning or displaying any message to the user.alert()
The browsers that support the alert()
method are:
- Chrome
- Edge
- Firefox
- Opera and also mobile browsers as well
JavaScript alert() and Cross-Site Scripting (XSS)
In the Ethical Hacking world, XSS is a very common vulnerability that hackers are searching for. A lot of them use the JavaScript alert() function to test a website against XSS vulnerabilities. You can learn more about this topic here.
Wrapping Up
We hope you were able to learn what JavaScript alert() is about and why it is used widely in web development. Since there are plenty of reasons to use alert()
is plenty, try out the examples and practice trying to use them for different purposes.
🔥 Learn JavaScript Programming – Beginner Friendly!
🔨 JavaScript Basics
👉 JavaScript alert()
👉 Difference between Let and Const
🔸 JavaScript Arrays
👉 JavaScript Array Filter
👉 JavaScript Array Find
👉 JavaScript forEach (Arrays)
👉 JavaScript Slice (Arrays)
👉 JavaScript Spread Operator
🔸 JavaScript Strings
👉 JavaScript Slice(Strings)
👉 JavaScript Includes
🌐 Web Development with JavaScript
👉 Store User Input in a variable with JS
⚙️ JavaScript Specifics
👉 Sorting Numbers in JS
👉 JavaScript Fetch API
👉 toLocaleDateString in JavaScript