If you came across some code with three dots in JavaScript in combination with arrays and you have no clue what that means, you came to the right place. I will give you a thorough explanation. The three dots in JavaScript represent the JavaScript Spread Operator and there are two use cases where they are used: Arrays and Objects.
In this article, we are looking at the JavaScript Spread Operator in array literals. Another separate article will cover spread in object literals.
The three dots in JavaScript, or the spread operator in array literals, was introduced with ES6, so if you have only worked with older versions of JavaScript, this might be your first encounter with the JavaScript Spread Operator.
👀 There are some related articles!
👉 JavaScript Slice (Arrays) – Made Easy
👉 JavaScript Array Filter – Made Easy
👉 JavaScript Array Find – Made Easy
Table of Contents
- What are the three dots used for in JavaScript arrays?
- The classic JavaScript method: concat()
- The ES6 spread in array literals method
- Why would this be useful?
- Conclusion
What are the three dots used for in JavaScript arrays?
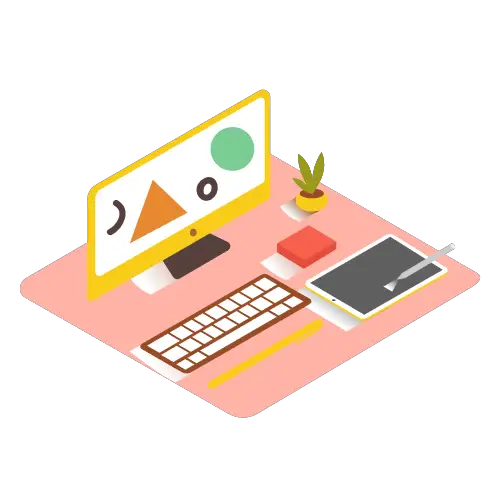
The three dots in JavaScript are used to concatenate two or multiple arrays. The spread operator was introduced to make this job easier, or better said, make the code shorter.
The classic JavaScript method: concat()
The classic method of combining two arrays in JavaScript was the concat()
method. Let’s say we have two arrays with car brands and we want to create another variable that combines those two arrays, so we know which cars are available for sale in our dealership.
let bmw = [ '330', '550', 'E36' ];
let audi = [ 'A4', 'A5', 'A6' ];
Code language: JavaScript (javascript)
To use the classic way, we would have to write something like this.
let availableCars = bmw.concat(audi);
console.log(availableCars);
Code language: JavaScript (javascript)
The resulting output would be:
[ '330', '550', 'E36', 'A4', 'A5', 'A6' ]
Code language: Bash (bash)
The ES6 spread in array literals method
Using the new spread method, it would look something like this:
let availableCars = [ ...bmw, ...audi ];
console.log(availableCars);
Code language: JavaScript (javascript)
With the resulting output of:
[ '330', '550', 'E36', 'A4', 'A5', 'A6' ]
Code language: Bash (bash)
Why would this be useful?
It becomes useful once we build functions with it. Let’s assume we want to choose a random car from the audi array and remove it from the array. After that, we want to update availableCars again to represent a list of remaining vehicles ready for sale in our store.
First, we need a function to choose a random car (we can also just choose a car we want to be removed, but let’s have a bit more fun here, try this on your own before looking up the solution below for a little challenge!).
The “choose a random item from the array” function
function choice(items) {
let idx = Math.floor(Math.random() * items.length);
return items[idx];
}
Code language: JavaScript (javascript)
This will return a random item from the array we pass to it.
Next, we need a function to slice the randomly selected car model out of our existing array and then add both arrays together, showing the remaining cars.
Using the spread method in array literals
function remove(item, items) {
for (let i = 0; i < items.length; i++) {
if (items[i] === item) {
return [ ...items.slice(0, i), ...items.slice(i + 1) ];
}
}
}
Code language: JavaScript (javascript)
Now we have to put it all together, call our functions and return our results.
function remove(item, items) {
for (let i = 0; i < items.length; i++) {
if (items[i] === item) {
return [ ...items.slice(0, i), ...items.slice(i + 1) ];
}
}
}
function choice(items) {
let idx = Math.floor(Math.random() * items.length);
return items[idx];
}
let bmw = [ '330', '550', 'E36' ];
let audi = [ 'A4', 'A5', 'A6' ];
let randomCar = choice(audi);
console.log(`The Random Car that is going to be removed is: ${randomCar}`);
let availableCars = remove(randomCar, audi);
let remainingCars = [ ...availableCars, ...bmw ];
console.log(remainingCars);
Code language: JavaScript (javascript)
The result of running this code is:
The Random Car that is going to be removed is: A5
[ 'A4', 'A6', '330', '550', 'E36' ]
Code language: Bash (bash)
Conclusion
As you can see, concatenating arrays back together using the spreadoperator method is easy. You don’t need to think too much about syntax because the three dots in JavaScript are easy to remember.
Understanding the JavaScript spreadoperator is fundamentally important when it comes to learning and understanding JavaScript. Every Job Interview will most likely ask you about this, so it’s good to have your JavaScript Spread Operator in check. I highly recommend practicing on your own using tools like Repl.it.
Make sure to check out more JavaScript Tutorials and learn all the basics on our website!