If you have recently started to learn Web Development, or in particular JavaScript, you might come across two different types of variables: Let and Const. Let and Const was introduced quite recently to replace the old Var variable assignment. If you are like me and you have started with a Web Development Course that is a bit dated and still uses Var for variable assignment, you will be baffled once you come across something that uses Let and Const. Gladly, itβs easy to explain the difference between Let and Const in Javascript.
Table of Contents
π₯ Learn JavaScript Programming β Beginner Friendly!
π¨ JavaScript Basics
π JavaScript alert()
πΒ Difference between Let and Const
πΈ JavaScript Arrays
πΒ JavaScript Array Filter
πΒ JavaScript Array Find
π JavaScript forEach (Arrays)
πΒ JavaScript Slice (Arrays)
πΒ JavaScript Spread Operator
πΈ JavaScript Strings
πΒ JavaScript Slice(Strings)
πΒ JavaScript Includes
π Web Development with JavaScript
πΒ Store User Input in a variable with JS
βοΈ JavaScript Specifics
πΒ Sorting Numbers in JS
π JavaScript Fetch API
π toLocaleDateString in JavaScript
What does Let and Const stand for?
Letβs first talk about what Let and Const actually mean. Let stands for βlettingβ and Const stands for βConstantβ. That alone should already give you a hint if it doesnβt let me break it down for you with an example.
// Dear computer, can you please
let
// this
day = "Wonderful"
Code language: JavaScript (javascript)
Which obviously translates to:
wonderful
Code language: Bash (bash)
Pretty logical, or isnβt it?
Const, on the other hand, explains itself pretty well. You use Const, or Constant for that matter, for variables that do not change. Let me give you another example.
let isSnowing = true
isSnowing = false
console.log(isSnowing)
Code language: JavaScript (javascript)
Can you guess what the output of this is? If you guessed false, you are right.
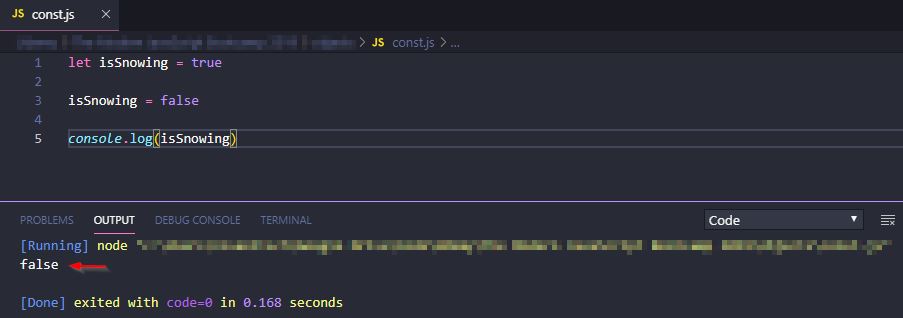
We first assign isSnowing
the boolean value of true, and then re-assign it the value false a line below. The ultimate result is false.
Now, what happens if we refactor this code to use Const instead of Let?
const isSnowing = true
isSnowing = false
console.log(isSnowing)
Code language: JavaScript (javascript)
We receive an error message:
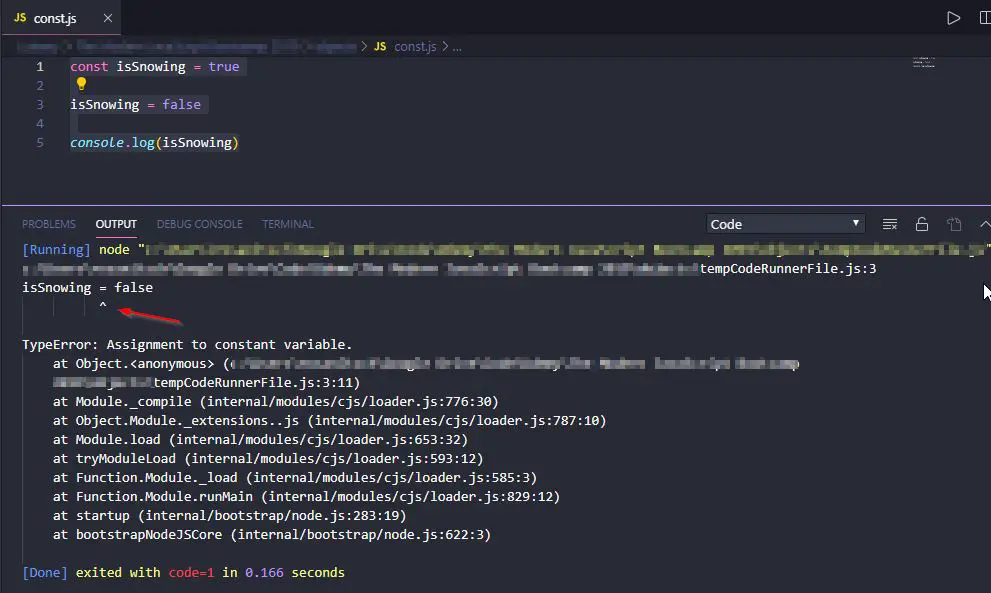
That means JavaScript knows that we are trying to modify a Constant variable so it wonβt run the code and throws an error at us. By now you probably can see some scenarios where this might be useful?
If we go ahead and comment out isSnowing
= false, our code will run:
const isSnowing = true
// isSnowing = false
console.log(isSnowing)
Code language: JavaScript (javascript)
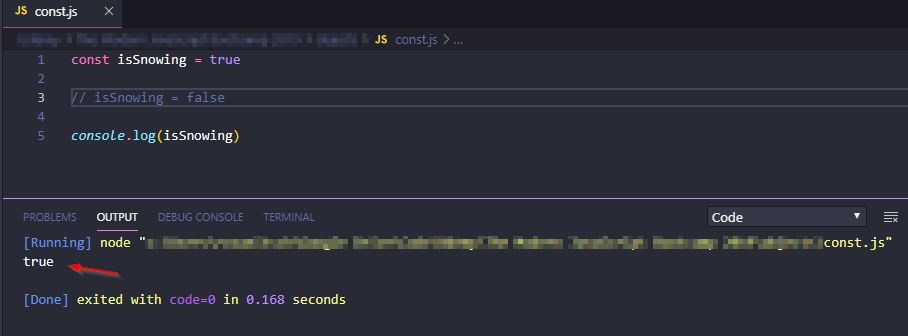
Why Const and Let were implemented?
If you have asked yourself this question by now, I got the answer to you: Readability.
To understand the difference between let and const, you have to simply think about the readability of code. If somebody else has to work on your code and sees a Const variable, he immediately knows that this is a fixed value and it is not going to change later on in the code, making it much easier for him or her to understand your code, whereas the opposite is true for Let.
Once I dug a little deeper it made perfect sense to me as well.