TypeScript is a popular programming language developed by Microsoft. It is a statically typed superset of JavaScript that compiles to plain JavaScript code. TypeScript adds optional static typing, classes, and interfaces to JavaScript, providing developers with a more structured and organized way to write code. In this TypeScript overview, we will dive into the benefits of using TypeScript, how it works, and its key features.
Table of Contents
- Static Checking in TypeScript
- Type Checking in TypeScript
- Why Use TypeScript?
- TypeScript Example
- Where to learn TypeScript?
- Conclusion
Static Checking in TypeScript
One of the key features of TypeScript is static checking. This means that TypeScript can catch errors in your code before it is run, as opposed to dynamic checking which is done at runtime in JavaScript.
Static checking ensures that your code is consistent and reliable and reduces the number of bugs and errors that might occur. This feature makes TypeScript ideal for large-scale projects, where maintaining code quality is essential.
Type Checking in TypeScript
Another important feature of TypeScript is type checking. Type checking ensures that your code is consistent with the expected types and reduces the likelihood of runtime errors.
In TypeScript, you can specify the type of a variable or function parameter using the syntax variableName: type
. This provides an extra layer of protection to your code, as TypeScript will warn you if you try to use a variable in a way that is not consistent with its type.
Why Use TypeScript?
TypeScript offers several benefits to developers that make it a popular choice for many projects. One of the main reasons is that it allows for better code organization and maintainability, making it easier to write and maintain large-scale applications. It also offers a smoother development experience, as errors are caught early in the development process, and autocomplete features help to speed up coding.
Another benefit of using TypeScript is that it is compatible with JavaScript, meaning you can easily integrate TypeScript into your existing JavaScript projects without starting from scratch. TypeScript also offers better documentation and tooling support, making it easier to debug and test code.
Key Features of TypeScript
Here are some of the key features of TypeScript:
- Statically typed: TypeScript provides static typing, allowing for early error detection and improved code consistency.
- Compatible with JavaScript: TypeScript is a superset of JavaScript, meaning you can use it with existing JavaScript code.
- Object-oriented: TypeScript supports classes and interfaces, making it ideal for object-oriented programming.
- Tooling support: TypeScript comes with various tools that help with code navigation, refactoring, and debugging.
TypeScript Example
Below you will find some TypeScript demo code to familiarize yourself with the syntax. Don’t worry; we will cover all of this later.
Defining a variable with a type:
let count: number = 5;
Code language: TypeScript (typescript)
In this example, we define a variable count
with the type number
.
Defining a function with a type:
function add(x: number, y: number): number {
return x + y;
}
Code language: TypeScript (typescript)
In this example, we define a function add
that takes two parameters of type number
and returns a value of type number
.
Defining an interface:
interface Person {
firstName: string;
lastName: string;
age: number;
}
Code language: TypeScript (typescript)
In this example, we define an interface Person
with three properties: firstName
and lastName
of type string
, and age
of type number
.
Defining a class:
class Car {
model: string;
make: string;
constructor(model: string, make: string) {
this.model = model;
this.make = make;
}
getCarDetails(): string {
return `This car is a ${this.make} ${this.model}.`;
}
}
Code language: TypeScript (typescript)
In this example, we define a class Car
with two properties model
and make
of type string
. We also define a constructor that takes two parameters of type string
and assigns them to the class properties. We also define a method getCarDetails
that returns a string with the car make and model.
Compiled TypeScript
If we look at an example of how the code above looks when it gets compiled into JavaScript, you will notice that it will get a lot shorter. This happens because things like type
or interface
doesn’t exist in vanilla JavaScript.
Left: TypeScript | Right: JavaScript |
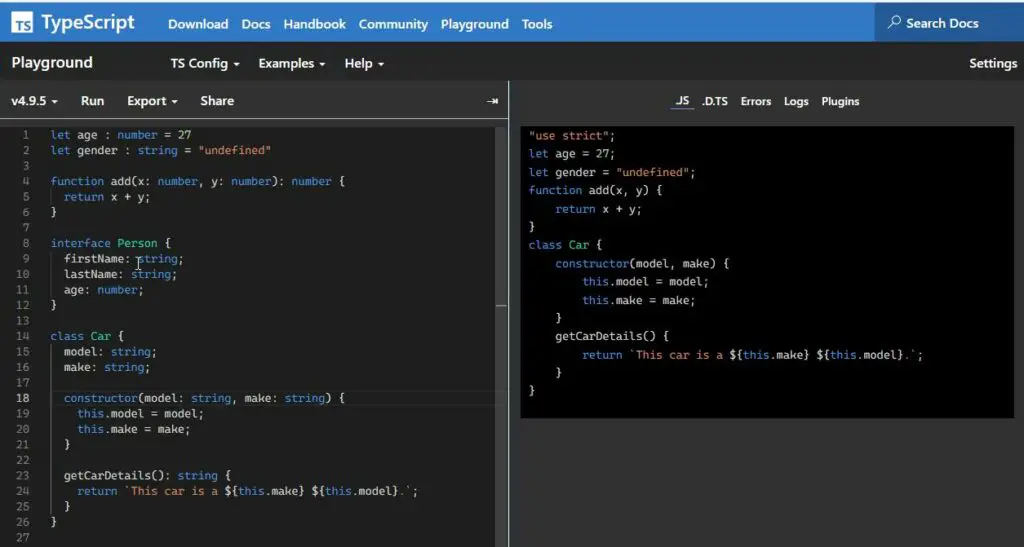
These are just a few basic examples of TypeScript syntax. There are many more features and concepts to explore in TypeScript, such as enums, unions, generics, and more.
Where to learn TypeScript?
If you’re interested in learning TypeScript, many resources are available online. The official TypeScript website provides a comprehensive guide to the language, including tutorials, documentation, and a playground where you can experiment with TypeScript code. Other popular resources include online courses, books, and YouTube tutorials.
If you are looking to learn TypeScript, I highly recommend checking out our TypeScript category. Our TypeScript category provides beginner-friendly TypeScript tutorials that include videos and follows a project-based approach using Replit. This makes it easier for you to learn and practice TypeScript in a hands-on way.
Whether you are new to TypeScript or have some experience with the language, ceos3c.com/typescript is a great resource that can help you improve your skills and knowledge. So, head over there and start your TypeScript learning journey today!
Conclusion
TypeScript is a powerful and popular programming language that offers a range of benefits to developers. With static checking, type checking, and a range of other features, it provides an organized and structured way to write code that is reliable and consistent.
In this TypeScript overview, we have covered the benefits of using TypeScript, how it works, and its key features. If you are interested in learning more about TypeScript, there are many resources available online that can help you get started with this powerful programming language.