After covering the bare basics of Astro JS by creating a very simple static site and hosting it on Netlify in the previous part of this tutorial series, it is time to move on. Now that you are comfortable with the folder structure and the basics of Astro, we can start to add some styling and dynamic content to our static site using Astro components.
First, we will talk about classic styling options using CSS. After this, in the next part of this series, we will move on from this and install Tailwind CSS in our Astro project for a better development experience. Using Tailwind CSS is completely optional but our preferred way of writing CSS.
After we learn how to apply some basic styling using CSS, we will start to add some dynamic content using Astro’s component script. This is another major step in understanding the Astro JS framework.
Table of Contents
- Astro JS Tutorial Series Part 2 Topics
- Styling Individual Pages with CSS
- Astro Component Structure
- Adding Dynamic Content
- Adding More Dynamic Content
- Summary
- Next Steps
Astro JS Tutorial Series Part 2 Topics
After finishing this part of the series, you will be able to:
- Style your Astro project with CSS.
- Work with Astro’s components and frontmatter.
- Add dynamic content to your Astro project.
You can clone the finished code of this series here. Make sure to checkout the 2-Styling-And-Dynamic-Content branch.
Styling Individual Pages with CSS
In Astro, we can simply add styles to individual pages by using the <style>
tags. This allows you to quickly add locally scoped styles to certain pages.
Let’s open our src/pages/about.astro
file. Currently, our about page looks pretty bland:
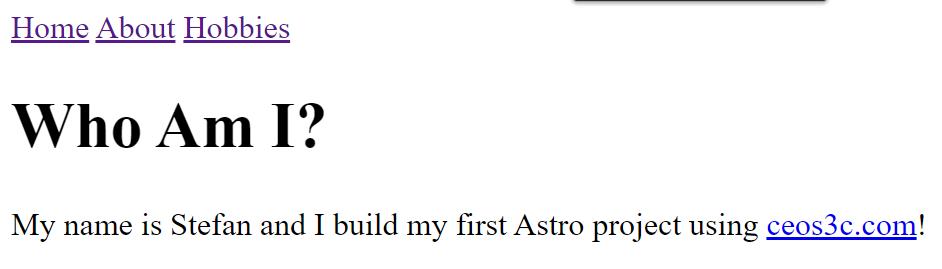
Ugh! Let’s add some styling to it:
<body>
<a href='/'>Home</a>
<a href='/about'>About</a>
<a href='/hobbies'>Hobbies</a>
<h1>Who Am I?</h1>
<p>
My name is Stefan and I build my first Astro project using <a
href='https://wwww.ceos3c.com'>ceos3c.com</a
> !
</p>
</body>
<style>
body {
font-family: sans-serif;
}
a {
color: orange;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
</style>
Code language: HTML, XML (xml)
Refresh your browser and look at the result. Nothing fancy, but it’s a start:
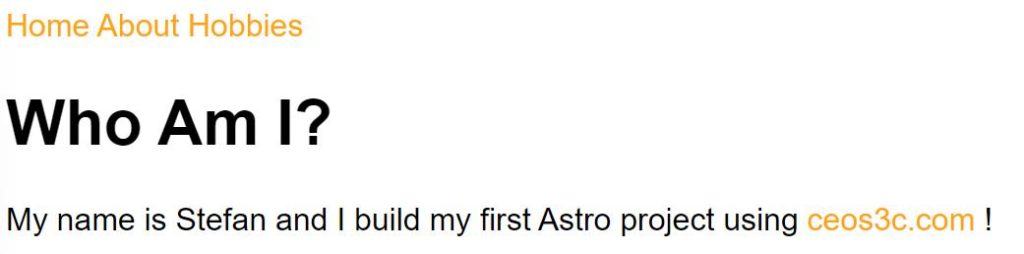
This way, you can quickly and easily apply local styles to your Astro pages. While we will be working with layouts, later on, knowing this is useful if you need to quickly add some CSS.
Astro Component Structure
Before we move on, it’s important that you understand how Astro component scripts work and how to use the frontmatter. Astro components consist of two parts:
- A Component Script
- A Component Template
---
Component Script (JavaScript)
---
Component Template (HTML and JS Expressions)
Code language: JavaScript (javascript)
Astro uses so-called code fences (the two lines of —- at the top of a .astro file) to separate the component script from your component template. If you are familiar with Markdown, you probably know these code fences as “frontmatter.”
Astro Component Script
The component script can be used to write JavaScript code that you want to use inside of your component template. If you have been working with other frameworks before: this is where your imports go, for example.
Let’s look at a simple example:
---
import MyComponent from '../components/MyComponent.astro'
---
<div>
<MyComponent />
</div>
Code language: JavaScript (javascript)
The Astro component script is where all your JavaScript code goes. You can use it for:
- Creating variables.
- Fetching data.
- Importing data from APIs or databases.
- Importing Astro components.
- Importing other supported frameworks like React or Vue.
The name “code fences” is no coincidence. These fences are used “to fence your code in” so that it won’t make it into your front-end application. The code written here is protected and will never get to the user’s browser.
Astro Component Template
The component template, on the other hand, is used for writing your HTML and JavaScript expressions. If you are familiar with JSX, this should come naturally. Let’s look at a simple example:
---
const favoriteColor = "Red"
---
<div>
<p> My favorite color is {favoriteColor}. </p>
</div>
Code language: JavaScript (javascript)
Output:
My favorite color is Red.
Adding Dynamic Content
Now that you have a basic understanding of how Astro components work, we can make parts of our project dynamic. All of this makes more sense later when we implement layouts and create multiple components. For now, we keep it to the basics.
Open the src/pages/index.astro
file and add the following code to it:
---
const pageTitle = "Landing Page"
---
<html lang='en'>
<head>
<meta charset='utf-8' />
<link rel='icon' type='image/svg+xml' href='/favicon.svg' />
<meta name='viewport' content='width=device-width' />
<meta name='generator' content={Astro.generator} />
<title>{pageTitle}</title>
</head>
<body>
<a href='/'>Home</a>
<a href='/about'>About</a>
<a href='/hobbies'>Hobbies</a>
<h1>{pageTitle}</h1>
</body>
</html>
Code language: JavaScript (javascript)
Refresh your browser and look at your page. It should now display “Landing Page”, both in the browser tab as well as in the <h1>
.
Instead of manually typing the page title in your HTML tags, you now know how to use variables to display dynamic text inside of your HTML.
Mini Challenge
Now go ahead and apply this knowledge to your src/pages/about.astro
and src/pages/hobbies.astro
files on your own:
- Add the variable
pageTitle
to the frontmatter. - Update your
<h1>
tags to display the page title using thepageTitle
variable.
Adding More Dynamic Content
Now that we know how to use variables and write JavaScript expressions in our HTML let’s make the src/pages/hobbies.astro
page more dynamic!
Add the following code between your code fences:
---
const pageTitle = "My Hobbies"
const hobbies = [
"Playing Video Games",
"Watching Movies",
"Playing Sports",
"Reading",
"Listening to Music",
]
---
Code language: JavaScript (javascript)
And then modify the HTML of your src/pages/hobbies.astro
to utilize the JavaScript map()
function to render your hobbies dynamically:
<body>
<a href='/'>Home</a>
<a href='/about'>About</a>
<a href='/hobbies'>Hobbies</a>
<h1>{pageTitle}</h1>
<ul>
{hobbies.map((hobby) => <li>{hobby}</li>)}
</ul>
</body>
Code language: JavaScript (javascript)
Now refresh your browser and check the result!
Summary
Now you should be able to style your Astro project using CSS, and you have also learned how to use JavaScript and Astro’s frontmatter to display data dynamically.
- You can use JavaScript expressions inside of your HTML between curly braces
{ }
. - You can use JavaScript code inside of your frontmatter.
- You can style Astro components with CSS using the
<style>
tag.
Next Steps
In the next part of this tutorial series, we are going to learn how to apply site-wide styling using Astro layouts, and we are going to install Tailwind CSS in our Astro project to make writing CSS easier.
Make sure to subscribe to our newsletter to get informed when a new part has been published.